- REST API
- Announcements
-
APIS list
- User
- Team
- Call
- Dialer Campaign
- Number
- Conversation Intelligence
- Content
- Message
-
Contact
- Contact overview
- List all Contacts
- Search Contacts
- Retrieve a Contact
- Create a Contact
- Update a Contact
- Delete a Contact
- Add phone number to a Contact
- Update a phone number from a Contact
- Delete a phone number from a Contact
- Add email to a Contact
- Update an email from a Contact
- Delete an email from a Contact
- Tag
- Webhook
- Company
- Integration
- Participant
- IVR Option
- A2P Campaign association
-
Webhooks
- Register an endpoint
- Webhook usage
- Payload
- User events
-
Call events
-
call.created
-
call.ringing_on_agent
-
call.agent_declined
-
call.answered
-
call.transferred
-
call.external_transferred
-
call.unsuccessful_transfer
-
call.hungup
-
call.ended
-
call.voicemail_left
-
call.commented
-
call.tagged
-
call.untagged
-
call.assigned
-
call.archived
-
call.hold
-
call.unhold
-
call.ivr_option_selected
-
call.comm_assets_generated
-
- Number events
- Message events
- Contact events
- Integration events
- Conversation Intelligence events
- Changelog
- Extras
Just like you, we are enthusiastic builders and we love to automate things. At Aircall, we are building the next-gen business phone system and we know that one of the best way to do that is to give you the ability to have the hands on Aircall features.
We are maintaining a Public API. This service allows any developers to retrieve and manage Aircall data with HTTP requests.
We are also supporting Webhooks. We can call back any endpoint of your choice, each time an event occurs on an Aircall account (contact added, call created, user switched off... see the Webhooks section for a complete list). Webhooks provide great scalability to your application and only require to set up a public web server!
Want to see an example of how easy it is to connect with Aircall Public API and Webhooks? Take a look at our tutorials!
Our Engineering team is on Twitter, follow them @aircalltech!
Check the Changelog if you've already implemented something based on previous versions of this documentation.
Not a Developer?
Install one of many integrations built by our partners in our Marketplace!
Subscribe to updates
Please subscribe to stay updated with the latest API and webhook updates.
REST API
Aircall Public API is a classic REST API. It can be used with any programming languages and offers a fast, reliable and secure access to an Aircall account information.
The root endpoint of Aircall Public API is https://api.aircall.io/v1
.
Please note that only HTTPS requests are valid as requests will communicate over SSL connection.
Each Public API request must be authenticated and should not exceed the rate limit, please check the Authentication and the rate limiting sections before jumping in our documentation!
RequestGET https://api.aircall.io
Response{ "resource": "Aircall Documentation", "contact": "support@aircall.io" }
Authentication
With Aircall Public API, Authentication can be done through OAuth or Basic Auth.
If you want to build an App for companies using Aircall - this is mainly the case for Technology Partners - please use the OAuth flow. It is a requirement to be listed on the Aircall App Marketplace. You can create a partner-specific account by signin up here!
If you are an Aircall customer, building for your own Aircall account only, the Basic Auth flow will do the trick.
All endpoints behave similarly between the two authentication methods, unless indicated otherwise in the documentation.
Aircall built a powerful Ecosystem of apps, providing its customers an easy way to enhance their voice experience.
In Aircall, integrations are enabled at the Company level, which means they can only be set by an Admin user. It is possible to configure several instances of an integration on one Aircall account.
Aircall implemented the OAuth 2.0 authentication protocol so you can easily build an app on top of Aircall and deploy it on Aircall's Marketplace.
Beyond the added security and scalability, the Aircall OAuth flow will make your app visible to all customers in the Aircall Dashboard and provide a simple way for customers to install your app from Aircall or from your own website.
Want to know every secret about OAuth? Read the official documentation RFC 6750!
-
Oauth terminology
-
install_uriStringAircall fetches the
install_uri
URI when starting the OAuth flow. This step is often used to display a Settings page, instructions on how to use the app or any useful information for the Admin.
It must eventually forward tohttps://dashboard.aircall.io/oauth/authorize
withclient_id
,redirect_uri
,response_type=code
andscope=public_api
query params. See Step 3 of the OAuth flow.Must use HTTPS. -
redirect_uriStringAircall sends the Admin's authorization
code
to theredirect_uri
URI, once they authorized the app, or the error if any.Must use HTTPS. -
client_idStringUsed in both the
[DASHBOARD] /oauth/authorize
and[API] /v1/oauth/token
request.Provided by Aircall. -
client_secretStringUsed in the request body of
[API] /v1/oauth/token
.Provided by Aircall. -
codeStringAn authentication code provided by Aircall valid for 10min.Must be converted in an
access_token
(see this endpoint). -
access_tokenStringToken used to send requests to Aircall Public API as a Company.More info on how to use it here.
-
stateStringAn optional string value created by your app to maintain state between the request and callback. This parameter should be used for preventing Cross-site Request Forgery and will be passed back to you, unchanged, in your
redirect_uri
.
OAuth credentials
Before starting building the OAuth flow for your app, you will need to get OAuth client_id
and client_secret
from Aircall. Contact us on marketplace@aircall.io, we will get back to you shortly with those credentials!
When signing up, an
install_uri
and a redirect_uri
will be asked, make sure you have them ready. Read the OAuth flow first to understand what they are!
OAuth flow
-
Aircall ﹣ When an Admin installs an App, a popup opens up and loads the
install_uri
URL. -
Partner ﹣ A Web server receives the
GET
request on theinstall_uri
route. This step is often used to display a custom Settings page, instructions on how to use the app and/or any useful information for the Admin. -
Partner ﹣ Once Step 2 is done, Web server redirects/forwards the request to the following Aircall URL:
https://dashboard.aircall.io/oauth/authorize?client_id=XX&redirect_uri=YY&response_type=code&scope=public_api&state=ZZ
-
Aircall ﹣ Aircall then displays the authorization window. Only users who are Admins will be able to see this window, others will be redirected to the Download page. Once the Admin consented to the permissions the app is requesting (
scope=public_api
), Aircall loads theredirect_uri
URL with acode
parameter. -
Partner ﹣ Web server receives the
GET
request on theredirect_uri
route with thecode
query param… -
Partner ﹣ …and sends a
[POST] https://api.aircall.io/v1/oauth/token
request to Aircall's Public API with the proper body params (see here). -
Aircall ﹣ Aircall authenticates the
[POST]
request, create anaccess_token
and sends it back to the Web server. -
Partner ﹣ Web server stores the
access_token
for further use!
Start the instructions at Step 3 if you want to trigger the install flow directly from your interface and not from the Aircall Dashboard.
Integrations can only be installed by users who are Admins on Aircall!
Check out our Ruby example app on Github to better understand how to implement the Aircall OAuth flow!
Get an access_token
via the Public API
Once you get an OAuth authorization code
from the OAuth flow, you need to convert it into a Public API access_token
with the following request.
This access_token
will then be used as a Bearer token in the Authorization
header of each Public API requests you will make and does not expire.
-
Body params
-
client_idStringThe
client_id
provided by Aircall. More information in the Oauth credentials section.Mandatory field. -
client_secretStringThe
client_secret
provided by Aircall. More information in the Oauth credentials section.Mandatory field. -
codeStringThe OAuth authorization code Aircall sent back to your server.Mandatory field.
-
redirect_uriStringYour
redirect_uri
.Mandatory field. -
grant_typeStringMust be
authorization_code
.Mandatory field.
RequestPOST https://api.aircall.io/v1/oauth/token { "client_id": YOUR_AIRCALL_CLIENT_ID, "client_secret": YOUR_AIRCALL_CLIENT_SECRET, "code": TMP_AUTHORIZATION_CODE, "redirect_uri": YOUR_REDIRECT_URI, "grant_type": "authorization_code" }
ResponseStatus: 200 OK { "access_token": "2d492d492d492d492d492d492d492d", "token_type": "Bearer", "created_at": 1585868617 }
Test the access_token
A /v1/ping endpoint is available to test the access_token
retrieved via the Public API (as described here).
The access_token
must be used in the Authorization
HTTP header of your request, as a Bearer token.
Requestcurl -X GET https://api.aircall.io/v1/ping \ -H "Authorization: Bearer {YOUR_ACCESS_TOKEN}"
ResponseStatus: 200 OK { "ping": "pong" }
As an Aircall customer, an api_id
and api_token
are needed to use Aircall Public API: go to your Company's Settings page. In the API Keys section, click on Add a new API key and get your api_token
and api_token
.
Do not forget to copy/paste your api_token
somewhere safe, we won't be able to retrieve it for you as Aircall does not store it in plain text!
If you are building an App for several companies using Aircall, please refer to the OAuth section.
Public API requests must be authenticated using HTTP Basic Authentication. The api_id
is the username and the api_token
is the password for each Public API requests.
The Authorization
HTTP header is constructed as follows:
- The
api_id
andapi_token
are concatenated with a single colon:
. - The resulting string is encoded using Base64.
- The authorization header results in
Authorization: Basic YOUR_ENCODED_STRING
.
Always prefer Base64 encoding in the
Authorization
HTTP header rather than the following: https://api_id:api_token@api.aircall.io.
It is not recommended to authenticate requests passing the api_id
and api_token
in the URL for security reasons and as some programming languages and platforms do not support it!
Requestcurl -X GET https://api.aircall.io/v1/ping \ -H "Authorization: Basic {YOUR_ENCODED_STRING}"
ResponseStatus: 200 OK { "ping": "pong" }
Rate limiting
Aircall limits the number of requests to its Public API to 60 requests per minute per company. The following headers are available in API headers' responses when the rate limit has been reached, giving more insight on your API usage:
header | description |
---|---|
X-AircallApi-Limit | Limit of requests for the API key used. |
X-AircallApi-Remaining | Remaining requests for this API key. |
X-AircallApi-Reset | Timestamp when the counter will be reset. |
Contact us on support.aircall.io if you need to make more requests per minute, we will add a higher rate limit to your API keys!
Pagination
When retrieving a list of objects with a [GET]
request, results are being paginated by Aircall.
Pagination information will be presented in the meta
object, available in the payload body and described below.
-
The meta object
-
countIntegerCount of items present in the current page.
-
totalIntegerCount of items in total, according to search params.
-
current_pageIntegerCurrent page number.
-
per_pageIntegerNumber of results retrieved per page.Default is 20. Minimum is 1, maximum is 50.
-
next_page_linkStringURL to follow to go to the next page results.Can be
null
. -
previous_page_linkStringURL to follow to go to the previous page results.Can be
null
.
The two following attributes can be set in any URL query params to navigate from one page to the other:
-
Query params
-
pageIntegerCurrent page.Default is 1.
-
per_pageIntegerNumber of results retrieved per pageDefault is 20.
Calls and Contacts are limited to 10,000 items, even with pagination on.
To pass over this limit, we encourage you to use the
from
param as much as you can!
Response{ "meta": { "count": 20, "total": 2234, "current_page": 1, "per_page": 20, "next_page_link": "https://api.aircall.io/v1/calls?page=2&per_page=20", "previous_page_link": null }, ... }
Errors
Aircall uses standard HTTP response codes to indicate the success or failure of an API request:
-
2xx
codes indicate success -
4xx
codes indicate an error that failed given the information provided -
5xx
codes indicate an error with Aircall's servers
An error
and a troubleshoot
key will be present in the response payload body. The troubleshoot
key is a readable description of the error.
More detailed HTTP response codes will be provided in endpoints documentation.
Response{ "error": "...", // Title "troubleshoot": "..." // Verbose message }
code | description |
---|---|
200 | OK - The request has succeeded. |
201 | Created - The request has been fulfilled and has resulted in one or more new resources being created. |
204 | No Content - Server has successfully fulfilled the request, no additional content sent in the response payload body. |
400 | Bad Request - Server cannot process the request due to something that is perceived to be a client error. |
403 | Forbidden - Lack of valid authentication credentials for the target resource. |
404 | Not Found - Server did not find the target resource. |
405 | Method Not Allowed - The method is known by the server but not supported. |
500 | Internal Server Error - Server encountered an unexpected condition. |
Content-Type
Every POST
, PUT
and DELETE
HTTP request sent to Aircall Public API must specify the Content-Type
entity header to application/json
.
RequestHTTP headers: { "Content-Type": "application/json" }
Announcements
Please refer this section for announcements related to deprecation or changes as well as availability of new APIs and Webhooks.
Title | Description | Changes effective from | Impacted/New Endpoints | Deprecation/Last Change Date |
---|---|---|---|---|
Deprecation of Webhook property id
|
The web hook id property has been replaced by the new property Webhook_id . Its recommended to use the same to retrieve, update and delete Webhooks. The property Webhook_id will be returned on creation of a Webhook |
01-07-2023 |
Retrieve a Webhook Update a Webhook Delete a Webhook |
15-04-2024 |
Deprecation of isAdmin Property during User Creation |
The isAdmin parameter is being deprecated from Create a User API and will no longer be available.
To assign Admin role to a User please use the role_ids parameter in the API
|
01-01-2024 | Create a User | 31-03-2024 |
New Call Webhook Events | Two new Webhook Call events are available i.e.
|
04-03-2024 | call.ivr_option_selected call.comm_assets_generated | N/A |
New Number Endpoints | One new Endpoint is available i.e.
|
05-15-2024 | Registration Status | N/A |
New Message Webhook Events | Three new Webhook message events are available i.e.
|
03-06-2024 | message.sent message.received message.status_updated | N/A |
New Message Endpoints | Four new Endpoints are available to use SMS/MMS capabilities via API i.e.
|
08-05-2024 | Fetch Number Configuration Create Number Configuration Delete Number Configuration Send message | N/A |
New Conversation Intelligence Event | New Conversation Intelligence event for Sentiment Analysis is available :
|
08-14-2024 | sentiment.created | N/A |
New Conversation Intelligence Event | New Conversation Intelligence event for Call Transcription is available:
|
08-26-2024 | transcription.created | N/A |
New Conversation Intelligence endpoints | Four new Endpoints are available to use Conversation Intelligence capabilities via API i.e.:
|
09-13-2024 | N/A | |
New Conversation Intelligence Event | New Conversation Intelligence events for Call Summary and Key Topics are available:
|
10-24-2024 |
summary.created topics.created |
N/A |
New Message Endpoint | One new Endpoint is available to use SMS capabilities capabilities via API i.e.:
|
11-25-2024 | N/A | |
New Call Webhook Event | New Webhook Call event is available i.e.:
|
02-18-2025 | N/A |
Contact us on support.aircall.io if you need more info regarding these announcements.
APIS list
In this section, we list all the available Public APIs.
User
Get all users associated to an Aircall account, or retrieve, create and update info about one specific User! You can also start outbound calls on an User's Phone app.
Users can be either Admins or Agents:
- Admins: can access the Dashboard and the Phone app, and can invite other users.
- Agents: can only access the Phone app and recording files.
Users are assigned to Numbers.
-
Attributes
-
idIntegerUnique identifier for the User.
-
direct_linkStringDirect API URL.
-
nameStringFull name of the User.Results of
first_name last_name
. -
emailStringEmail of the User.
-
created_atStringTimestamp when the User was created, in UTC.
-
availableBooleanCurrent availability status of the User, based on their working hours.
-
availability_statusStringCurrent working status of the User.Can be
available
,custom
(= available according to their Working Hours and Timezone) orunavailable
(= Do Not Disturb or other unavailable status). More availablility statuses can be retrieved, see the Availability table below. -
substatusStringCurrent substatus of the User.If user selects availability_status as
available
orcustom
(available according to working hours or timezone) then substatus will bealways_open
.
If user selects availability_status asunavailable
and doesn't select a substatus or unavailability reason in Phone App then it will bealways_closed
.
If user selects availability_status asunavailable
and selects a substatus or unavailability reason in Phone App i.e.Out for lunch
,On a break
,In training
,Back office
orOther
. The substatus will contain the exact substauts or unavailability reason selected. -
numbersArrayList of Numbers associated to this User.
-
time_zoneStringThe User's timezone. This can be set either from the Dashboard or the Phone (check our Knowledge Base).Default is
Etc/UTC
. More details on Timezones here. -
languageStringThe User's preferred language. This can be set either from the Dashboard or the Phone (check our Knowledge Base).The format is IETF language tag. Default is
en-US
. -
wrap_up_timeIntegerA pre-set timer triggered after a call has ended, during which the user can’t receive any calls. Learn more..
-
Availability
-
available
StringAgent ready to answer calls. -
offline
StringAgent not online. -
do_not_disturb
StringAgent toggled themself as do not disturb. -
in_call
StringAgent is currently on a call. -
after_call_work
StringAgent is performing their after-call work (tagging a call or wrapping up).
Find a proper definition of each of those availability statuses in our Knowledge Base
and retrieve those granular availability statuses with this endpoint!
EndpointsGET /v1/usersList all Users GET /v1/users/:idRetrieve a User POST /v1/usersCreate a User PUT /v1/users/:idUpdate a User DELETE /v1/users/:idDelete a User GET /v1/users/availabilitiesRetrieve list of Users availability GET /v1/users/:id/availabilityCheck availability of a User POST /v1/users/:id/callsStart an outbound call POST /v1/users/:id/dialDial a phone number in the Phone
Fetch all Users associated to a Company and their information.
Looking for more granular availability statuses? Check the availability endpoint!
-
Query params
-
fromStringSet a minimal creation date for Users (UNIX timestamp).
-
toStringSet a maximal creation date for Users (UNIX timestamp).
-
orderStringReorder entries by
created_at
. Can beasc
ordesc
.Default value isasc
Pagination params can be used on this request.
RequestGET /v1/users
ResponseStatus: 200 OK { "meta": { "count": 3, "total": 3, "current_page": 1, "per_page": 20, "next_page_link": null, "previous_page_link": null }, "users": [ { "id": 456, "direct_link": "https://api.aircall.io/v1/users/456", "name": "John Doe", "email": "john.doe@aircall.io", "available": true, "availability_status": "available", "created_at": "2019-12-29T10:03:18.000Z", "time_zone": "America/New_York", "language": "en-US", "substatus": "always_opened", "wrap_up_time": 0 }, { "id": 457, "direct_link": "https://api.aircall.io/v1/users/457", "name": "Amy Rudd", "email": "amy.rudd@aircall.io", "available": true, "availability_status": "custom", "created_at": "2019-12-30T18:10:21.000Z", "time_zone": "Etc/UTC", "language": "en-US", "substatus": "always_opened", "wrap_up_time": 0 }, { "id": 458, "direct_link": "https://api.aircall.io/v1/users/458", "name": "Vera Martin", "email": "vera.martin@aircall.io", "available": false, "availability_status": "unavailable", "created_at": "20120-01-02T09:01:58.000Z", "time_zone": "Europe/Paris", "language": "fr-FR", "substatus": "always_opened", "wrap_up_time": 0 } ] }
Retrieve details of a specific User.
Looking for more granular availability statuses for a User? Check this dedicated endpoint.
-
Path params
-
idIntegerUnique identifier for the User.
RequestGET /v1/users/:id
ResponseStatus: 200 OK { "user": { "id": 456, "direct_link": "https://api.aircall.io/v1/users/456", "name": "John Doe", "email": "john.doe@aircall.io", "available": true, "availability_status": "available", "created_at": "2019-12-29T10:03:18.000Z", "time_zone": "America/New_York", "language": "en-US", "substatus": "always_opened", "wrap_up_time": 0, "default_number_id": 1234, "numbers": [ { "id": 1234, "direct_link": "https://api.aircall.io/v1/numbers/1234", "name": "French Office", "digits": "+33 1 76 11 11 11", "created_at": "2020-01-02T11:41:01.000Z", "country": "FR", "time_zone": "Europe/Paris", "open": true, "availability_status": "custom", "is_ivr": true, "live_recording_activated": true, "priority": null, "messages": { "welcome": "https://example.com/welcome.mp3", "waiting": "https://example.com/waiting_music.mp3", "ivr": "https://example.com/ivr_message.mp3", "voicemail": "https://example.com/voicemail.mp3", "closed": "https://example.com/closed_message.mp3", "callback_later": "https://example.com/callback_later.mp3", "unanswered_call": "https://example.com/unanswered_call.mp3", "after_hours": "https://example.com/closed_message.mp3", "ringing_tone": "https://example.com/waiting_music.mp3" } } ] } }
Users can be created one at a time. After creation, a unique user ID will be included in the response's payload. An invitation email will be sent to the User, they will have to confirm their account before being able to use Aircall.
A
user.created
Webhook event is sent on User creation. More information in the Webhooks section.
-
Body params
-
emailStringMust be a valid and unique email.Mandatory field.
-
first_nameStringCan't be blank if defined.Mandatory field.
-
last_nameStringCan't be blank if defined.Mandatory field.
-
availability_statusString
available
,custom
orunavailable
.Default value isavailable
-
role_idsArray<String>
owner
,supervisor
,admin
oragent
.Default value isagent
-
wrap_up_timeIntegerOptional number of seconds as wrap-up time after a call. It applies only to a user that includes the role
agent
Default value is0
seconds
code | description |
---|---|
422 | Mandatory attribute missing, check the troubleshoot field. |
RequestPOST /v1/users { "email": "jeffrey.curtis@aircall.io", "first_name": "Jeffrey", "last_name": "Curtis" }
ResponseStatus: 201 Created { "user": { "id": 458, "direct_link": "https://api.aircall.io/v1/users/458", "name": "Jeffrey Curtis", "email": "jeffrey.curtis@aircall.io", "available": false, "availability_status": "available", "created_at": "2020-02-18T20:52:22.000Z", "time_zone": "Etc/UTC", "language": "en-US", "numbers": [], "wrap_up_time": 0 } }
Some fields can be updated via the Public API. For instance, a User's availability status could be automatically updated based on information from their calendar or a workforce management tool.
-
Path params
-
idIntegerUnique identifier for the User.
-
Body params
-
first_nameStringCan't be blank if defined.
-
last_nameStringCan't be blank if defined.
-
availability_statusString
available
,custom
orunavailable
. -
role_idsArray<String>
owner
,supervisor
,admin
oragent
. -
wrap_up_timeIntegerNumber of seconds as wrap-up time after a call. It applies only to a user that has the role
agent
.
RequestPUT /v1/users/:id { "first_name": "Jeff Updated" }
ResponseStatus: 200 OK { "user": { "id": 458, "direct_link": "https://api.aircall.io/v1/users/458", "name": "Jeff Updated Curtis", "email": "jeffrey.curtis@aircall.io", "available": false, "availability_status": "available", "created_at": "2020-02-18T20:52:22.000Z", "time_zone": "America/New_York", "language": "en-US", "numbers": [], "wrap_up_time": 55 } }
When deleting a User, all data associated to them will also be destroyed and won't be recoverable.
A
user.deleted
Webhook event is sent on User deletion. More information in the Webhooks section.
-
Path params
-
idIntegerUnique identifier for the User.
code | description |
---|---|
204 | User will be deleted in the next minutes, depending on how many calls and data are associated to them. |
422 | Server unable to process the request, error will be described in the troubleshoot field. |
RequestDELETE /v1/users/:id
ResponseStatus: 204 No Content
List the detailed availability of all Users, displayed in the Dashboard's Activity Feed.
Please refer to the User object definition to see all the possible values of the availability
field.
Find a detailed definition of each availability status in our Knowledge Base.
-
Query params
-
fromStringSet a minimal creation date for Users (UNIX timestamp).
-
toStringSet a maximal creation date for Users (UNIX timestamp).
-
orderStringReorder entries by
created_at
. Can beasc
ordesc
.Default value isasc
RequestGET /v1/users/availabilities
ResponseStatus: 200 OK { "meta": { "count": 3, "total": 3, "current_page": 1, "per_page": 20, "next_page_link": null, "previous_page_link": null }, "users": [ { "id": 456, "availability": "available" }, { "id": 457, "availability": "offline" } { "id": 458, "availability": "do_not_disturb" } ] }
Retrieve the detailed availability of a specific User, displayed in the Dashboard's Activity Feed.
Please refer to the User object definition to see all the possible values of the availability
field.
Find a proper definition of each availability status in our Knowledge Base.
-
Path params
-
idIntegerUnique identifier for the User.
RequestGET /v1/users/:id/availability
ResponseStatus: 200 OK { "availability": "after_call_work" }
Outbound calls can be automatically started for a User from their Phone app. It is often used to build a Click-to-call feature!
A Number ID and a phone number to dial must be provided in the request's body.
The User must be available, not on a call and associated to the Number.
Number must be active (validated), inactive numbers can be seen in the Aircall Dashboard.
This feature is only available on Aircall Phone app on Desktop for now, not yet on iOS and Android.
Please note, the API doesn’t support multiple sessions. It is not recommended to use this API along with Aircall CTI or Web App when multiple tabs are open
-
Path params
-
idIntegerUnique identifier for the User.
-
Body params
-
number_idIntegerUnique identifier of the Number to use for the call.Mandatory field.
-
toStringThe number to dial in E.164 format.Mandatory field.
code | description |
---|---|
204 | Success. |
400 | Number not found or invalid number to dial. |
405 | User not available. |
RequestPOST /v1/users/:id/calls { "number_id": 123, "to": "+18001231234" }
ResponseStatus: 204 No Content
Sending a request to this endpoint will fill the User's Phone app with the to
params. They will then be able to start the call within the Phone app and will be free to choose from which Number they want to start the call from. It is often used to build a Click-to-dial feature!
User must be available and not on a call.
This feature is also available from the Aircall Everywhere CTI.
This feature is only available on Aircall Phone app on Desktop for now, not yet on iOS and Android.
-
Path params
-
idIntegerUnique identifier for the User.
-
Body params
-
toStringThe phone number to dial in E.164 format.Mandatory field.
code | description |
---|---|
204 | Success. |
400 | Invalid number to dial. |
405 | User not available. |
RequestPOST /v1/users/:id/dial { "to": "+18001231234" }
ResponseStatus: 204 No Content
Team
Users can be grouped in Teams and managed from the Dashboard.
Teams are only used in call distributions of Numbers.
More info on how to use Aircalls' Team feature in our Knowledge Base.
-
Attributes
-
idIntegerUnique identifier for the Team.
-
direct_linkStringDirect API URL.
-
nameStringFull name of the Team.
name
must be unique in a company and the length of the string should 64 characters maximum. -
created_atStringTimestamp when the Team was created, in UTC.
-
usersArrayList of Users associated to this Team.User attributes
available
andavailability_status
are not available in Teams endpoint.
Fetch all Teams associated to a company and their information.
-
Query params
-
orderStringReorder entries by
created_at
. Can beasc
ordesc
.Default value isasc
Pagination params can be used on this request.
code | description |
---|---|
200 | Success. |
403 | Forbidden. Invalid API key or Bearer access token |
RequestGET /v1/teams
ResponseStatus: 200 OK { "meta": { "count": 1, "total": 1, "current_page": 1, "per_page": 20, "next_page_link": null, "previous_page_link": null }, "teams": [ { "id": 678, "name": "Global Sales", "direct_link": "https://api.aircall.io/v1/teams/678", "created_at": "2020-03-10T08:31:43.000Z", "users": [ { "id": 456, "direct_link": "https://api.aircall.io/v1/users/4c56", "name": "John Doe", "email": "john.doe@aircall.io", "created_at": "2019-12-29T10:03:18.000Z", "time_zone": "America/New_York" }, { "id": 457, "direct_link": "https://api.aircall.io/v1/users/457", "name": "Amy Rudd", "email": "amy.rudd@aircall.io", "created_at": "2019-12-30T18:10:21.000Z", "time_zone": "Etc/UTC" } ] } ] }
Retrieve details of a specific Team.
-
Path params
-
idIntegerUnique identifier for the Team.
code | description |
---|---|
200 | Success. |
403 | Forbidden. Invalid API key or Bearer access token |
404 | Not found. Id does not exist |
RequestGET /v1/teams/:id
ResponseStatus: 200 OK { "team": { "id": 678, "name": "Global Sales", "direct_link": "https://api.aircall.io/v1/teams/678", "created_at": "2020-03-10T08:31:43.000Z", "users": [ { "id": 456, "direct_link": "https://api.aircall.io/v1/users/4c56", "name": "John Doe", "email": "john.doe@aircall.io", "created_at": "2019-12-29T10:03:18.000Z", "time_zone": "America/New_York" }, { "id": 457, "direct_link": "https://api.aircall.io/v1/users/457", "name": "Amy Rudd", "email": "amy.rudd@aircall.io", "created_at": "2019-12-30T18:10:21.000Z", "time_zone": "Etc/UTC" } ] } }
A name must be provided when creating a Team.
Please use the following endpoint to add Users to it.
-
Body params
-
nameStringCan't be blank if defined.Mandatory field.
code | description |
---|---|
201 | Success. |
400 | Company might have reached the maximum number of teams allowed on their plan. Name must be smaller than 64 characters and unique in the company. |
403 | Forbidden. Invalid API key or Bearer access token |
RequestPOST /v1/teams { "name": "Support USA" }
ResponseStatus: 201 Created { "team": { "id": 679, "name": "Support USA", "direct_link": "https://api.aircall.io/v1/teams/679", "created_at": "2020-03-10T20:29:52.000Z", "users": [] } }
When deleting Teams, they will be removed from the Numbers call distribution.
Users and Calls won't be deleted.
-
Path params
-
idIntegerUnique identifier for the Team.
code | description |
---|---|
204 | Success. |
403 | Forbidden. Invalid API key or Bearer access token |
404 | Not found. Id does not exist |
422 | Server unable to process the request, error will be described in the troubleshoot field. |
RequestDELETE /v1/teams/:id
ResponseStatus: 200 OK
Users can be added one by one to a Team.
-
Path params
-
team_idIntegerUnique identifier for the Team.
-
user_idIntegerUnique identifier for the User.
code | description |
---|---|
201 | Success. |
403 | Forbidden. Invalid API key or Bearer access token |
404 | Not found. Either team_id or user_id does not exist |
422 | Server unable to process the request, error will be described in the troubleshoot field. |
RequestPOST /v1/teams/:team_id/users/:user_id
ResponseStatus: 201 Created { "team": { "id": 679, "name": "Support USA", "direct_link": "https://api.aircall.io/v1/teams/679", "created_at": "2020-03-10T20:29:52.000Z", "users": [ { "id": 456, "direct_link": "https://api.aircall.io/v1/users/4c56", "name": "John Doe", "email": "john.doe@aircall.io", "created_at": "2019-12-29T10:03:18.000Z", "time_zone": "America/New_York" } ] } }
Users can be delete one by one from a Team.
Calls made and received by this User won't be deleted.
-
Path params
-
team_idIntegerUnique identifier for the Team.
-
useridIntegerUnique identifier for the User.
code | description |
---|---|
200 | Success. |
403 | Forbidden. Invalid API key or Bearer access token |
404 | Not found. Either team_id or user_id does not exist |
422 | Server unable to process the request, error will be described in the troubleshoot field. |
RequestDELETE /v1/teams/:team_id/users/:user_id
ResponseStatus: 200 OK { "team": { "id": 679, "name": "Support USA", "direct_link": "https://api.aircall.io/v1/teams/679", "created_at": "2020-03-10T20:29:52.000Z", "users": [] } }
Call
Calls are an essential part of how Aircall users interact with the product. Our mission is to give real-time insights to make the call experience as delightful as possible!
There are three types of calls:
- Inbound calls: where an external person reaches an Agent.
- Outbound calls: where an Agent starts a call from their Phone app to reach an external person.
- Internal calls: where an Agent calls another Agent.
More information available on each type of Call at the bottom of this section.
Please refer to the User object if you are looking to start an outbound call or to dial a phone number!
-
Attributes
-
idIntegerUnique identifier for the Call.Please note, Call id Data Type is
Int64
-
sidStringCall identifier from call providerPlease note
sid
attribute refers tocall_uuid
and has same value ascall_uuid
.sid
is only available in Call APIs -
call_uuidStringCall identifier from call providerPlease note
call_uuid
attribute refers tosid
and has same value assid
.call_uuid
is only available in Call Webhook events -
direct_linkStringDirect API URL.
-
started_atIntegerUNIX timestamp when the Call started, in UTC.
-
answered_atIntegerUNIX timestamp when the Call has been answered, in UTC.
-
ended_atIntegerUNIX timestamp when the Call ended, in UTC.
-
durationIntegerDuration of the Call in seconds.This field is computed by
ended_at - started_at
. -
statusStringCurrent status of the Call.Can be initial, answered or done.
-
directionStringDirection of the Call.Could be inbound or outbound.
-
raw_digitsStringInternational format of the number of the caller or the callee. For an
anonymous
call, the value isanonymous
. -
assetStringIf present, a secured webpage containing the voicemail or live recording for this Call.URL format is
https://assets.aircall.io/[recording,voicemail]/:call_id
. -
recordingStringIf present, the direct URL of the live recording (mp3 file) for this Call. This feature can be enabled from the Aircall Dashboard, on each Number - more information in our Knowledge Base.This link is valid for 1 hour only.
-
recording_short_urlStringWhen
recording
is present, a unique short URL redirecting to it.This link is valid for 3 hours only.
URL format ishttps://short-urls.aircall.io/v1/:uuid
. -
voicemailStringOnly present if a voicemail was left. Voicemails can only be left by callers on inbound calls. If present, the direct URL of a voicemail (mp3 file) for this Call.This link is valid for 1 hour only.
-
voicemail_short_urlStringWhen
voicemail
is present, a unique short URL redirecting to it.This link is valid for 3 hours only.
URL format ishttps://short-urls.aircall.io/v1/:uuid
. -
archivedBooleanDescribe if Call needs follow up.
-
missed_call_reasonStringRepresenting the reason why the Call was missed.Can be
out_of_opening_hours
,short_abandoned
,abandoned_in_ivr
,abandoned_in_classic
,no_available_agent
oragents_did_not_answer
. -
costStringCost of the Call in U.S. cents.Cost is a legacy field which has been deprecated, we might reintroduce it in the future. For more info please reach out to us on support.aircall.io.
-
numberObjectFull Number object attached to the Call.
-
userObjectFull User object who took or made the Call.
-
contactObjectFull Contact object attached to the Call.
-
assigned_toObjectFull User object assigned to the Call.
-
teamsArrayFull Teams object assigned to the Call.Teams are only assigned to inbound calls.
-
transferred_byObjectUser who performed the Call transfer.
-
transferred_toObjectUser to whom the Call was transferred to.If Call is transferred to a Team, this field will represent the first User of the Team. It won't be available in case of external call transfers.
-
external_transferred_toStringExternal number to which the Call was transferred to.Only available via call.external_transferred event
-
commentsArrayNotes added to this Call by Users.
-
tagsArrayTags added to this Call by Users.
-
participantsArrayParticipants involved in a conference call.Please note participants attribute is referred as
conference_participants
in Call APIs and asparticipants
in call Webhook events -
ivr_options_selectedArrayIVR options selected in a smartflow enabled number.ivr_options_selected is retrievable via Call APIs using fetch timeline query param
1. Inbound calls
Inbound calls are initiated by an external person, calling an Aircall Number. Once started, calls will follow the Call distribution tree defined in the Aircall Dashboard (see our Knowledge Base).
If an Inbound call is not answered, it is then considerred as missed. All missed calls will have the missed_call_reason
field defined in their payload, and the answered_at
field will be null
.
The duration
field is computed by the following: ended_at - started_at
. As the ringing time is included in it, use the ended_at - answered_at
calculation to get the talking time of the call!
More info on Inbound calls lifecycle in the Webhook section.
2. Outbound calls
Outbound calls are initiated by Agents from their Phone app, calling an external person.
If an Outbound call is not answered by the external person, the answered_at
field will be null
.
The duration
field is computed by the following: ended_at - started_at
. As the ringing time is included in it, use the ended_at - answered_at
calculation to get the talking time of the call. If an outbound call is answered by a voicemail, it will be considerred as answered (Aircall does not support Answering Machine Detection yet).
More info on Outbound calls lifecycle in the Webhook section.
Starting an outbound call from an Agent's Phone is feasible
with the Start Outbound Call endpoint, defined on the User object.
3. Internal calls
Internal calls are initiated by an Agent, calling another Agent. Those calls are not rendered in the Public API.
More info on how to use Intercall calls in our Knowledge Base.
Call Comments (also called Notes)
Calls can be commented by Agents or via the Public API endpoint [POST] /v1/calls/:id/comments
.
Here is the object description of a comment:
-
Attributes
-
idIntegerUnique identifier for the Comment.
-
contentStringContent of the Comment, written by Agent or via Public API.
-
posted_atStringTimestamp of when the Comment was created.
-
posted_byObjectUser object who created the Comment.Will be
null
if Comment is posted via Public API.
EndpointsGET /v1/callsList all Calls GET /v1/calls/:idRetrieve a Call GET /v1/calls/searchSearch Calls POST /v1/calls/:id/transfersTransfer a Call POST /v1/calls/:id/commentsComment a Call POST /v1/calls/:id/tagsTag a Call PUT /v1/calls/:id/archiveArchive a Call PUT /v1/calls/:id/unarchiveUnarchive a Call POST /v1/calls/:id/pause_recordingPause recording on a Call POST /v1/calls/:id/resume_recordingResume recording on a Call DELETE /v1/calls/:id/recordingDelete Call recording DELETE /v1/calls/:id/voicemailDelete Call voicemail POST /v1/calls/:id/insight_cardsInsight Cards GET /v1/calls/:call_id/transcriptionRetrieve a transcription GET /v1/calls/:call_id/sentimentsRetrieve sentiments GET /v1/calls/:call_id/topicsRetrieve topics GET /v1/calls/:call_id/summaryRetrieve a summary
Fetch all Calls associated to a company and their information.
By default, Calls are ordered by ascending IDs - consider using the order=desc
query param if you want to get the last calls made by an account!
Only three months of history is available. Please contact our Support team on support.aircall.io to get a one-time export of calls.
By default contact details are not added in response payload. To add the contact details in response set fetch_contact to
true
in query parameters.
-
Query params
-
fromStringSet a minimal creation date for Calls (UNIX timestamp).
-
toStringSet a maximal creation date for Calls (UNIX timestamp).
-
orderStringReorder entries by
created_at
. Can beasc
ordesc
.Default value isasc
-
fetch_contactBooleanWhen set to
true
, adds contacts details in response. -
fetch_short_urlsBooleanWhen set to
true
, adds short urls in response. -
fetch_call_timelineBooleanWhen set to
true
, it will ivr_options_selected at the same level of the call
Using the pagination system, you can retrieve up to 10,000 Calls. To pass over this limit, we encourage you to use the
from
param as much as you can!
RequestGET /v1/calls
ResponseStatus: 200 OK { "meta": { "count": 20, "total": 2234, "current_page": 1, "per_page": 20, "next_page_link": "https://api.aircall.io/v1/calls?order=asc&page=2&per_page=20", "previous_page_link": null }, "calls": [ { "id": 812, "sid": "CA1234567890", "direct_link": "https://api.aircall.io/v1/calls/812", "direction": "outbound", "status": "done", "missed_call_reason": null, "started_at": 1584998199, "answered_at": 1584998205, "ended_at": 1584998210, "duration": 11, "voicemail": null, "recording": null, "asset": null, "raw_digits": "+1 800-123-4567", "user": { "id": 456, "direct_link": "https://api.aircall.io/v1/users/456", "name": "John Doe", "email": "john.doe@aircall.io", "available": true, "availability_status": "available", "created_at": "2019-12-29T10:03:18.000Z", "time_zone": "America/New_York", "language": "en-US" }, "contact": null, "archived": false, "assigned_to": null, "transferred_by": null, "transferred_to": null, "cost": "2.34", "number": { "id": 1234, "direct_link": "https://api.aircall.io/v1/numbers/1234", "name": "French Office", "digits": "+33 1 76 11 11 11", "created_at": "2020-01-02T11:41:01.000Z", "country": "FR", "time_zone": "Europe/Paris", "open": true, "availability_status": "custom", "is_ivr": true, "live_recording_activated": true, "priority": null, "messages": { ... } }, "comments": [ { "id": 735, "content": "Please call back this customer!", "posted_at": 1587994808, "posted_by": { "id": 456, "direct_link": "https://api.aircall.io/v1/users/456", "name": "Johnn Doe", "email": "john.doe@aircall.io", "available": true, "availability_status": "available", "created_at": "2019-12-29T10:03:18.000Z" } }, ... ], "tags": [ { "id": 678, "name": "General Inquiries", "created_at": 1587995020, "tagged_by": { "id": 456, "direct_link": "https://api.aircall.io/v1/users/456", "name": "Johnn Doe", "email": "john.doe@aircall.io", "available": true, "availability_status": "available", "created_at": "2019-12-29T10:03:18.000Z" } }, ... ], "teams": [], "ivr_options_selected": [ { "id": "5cb41cde-aed2-4357-a98c-e1b33d68851a", "title": "", "key": "1", "branch": "Default", "created_at": "2024-10-01T06:54:45.556Z", "transition_started_at": "2024-10-01T06:54:35.332Z", "transition_ended_at": "2024-10-01T06:54:45.513Z" } ] }, { "id": 813, "direct_link": "https://api.aircall.io/v1/calls/813", "direction": "inbound", "status": "done", "missed_call_reason": null, "started_at": 1584998199, "answered_at": "no_available_agent", "ended_at": 1584998210, "duration": 22, "voicemail": null, "recording": null, "asset": null, "raw_digits": "+1 800-123-4567", "user": null, "contact": null, "archived": false, "assigned_to": null, "transferred_by": null, "transferred_to": null, "cost": "0", "number": { "id": 1234, "direct_link": "https://api.aircall.io/v1/numbers/1234", "name": "French Office", "digits": "+33 1 76 11 11 11", "created_at": "2020-01-02T11:41:01.000Z", "country": "FR", "time_zone": "Europe/Paris", "open": true, "availability_status": "custom", "is_ivr": true, "live_recording_activated": true, "priority": null, "messages": { ... } }, "comments": [...], "tags": [...], "teams": [ { "id": 678, "name": "Global Sales", "direct_link": "https://api.aircall.io/v1/teams/678", "created_at": "2020-03-10T08:31:43.000Z" } ], "ivr_options_selected": [ { "id": "5cb41cde-aed2-4357-a98c-e1b33d68851a", "title": "", "key": "1", "branch": "Default", "created_at": "2024-10-01T06:54:45.556Z", "transition_started_at": "2024-10-01T06:54:35.332Z", "transition_ended_at": "2024-10-01T06:54:45.513Z" } ] } ... ] }
Use this endpoint to asynchronously retrieve a Call data like duration, direction, status, timestamps, comments or tags…
To get Calls information in real time, we recommend using Calls Webhooks events - for instance to log information automatically in your database after a Call. By default contact details are not added in response payload. To add the contact details in response set fetch_contact to
true
in query parameters.
-
Path params
-
idIntegerUnique identifier for the Call.
-
fetch_contactBooleanWhen set to
true
, adds contacts details in response. -
fetch_short_urlsBooleanWhen set to
true
, adds short urls in response. -
fetch_call_timelineBooleanWhen set to
true
, it will return ivr_options_selected at the same level of the call
RequestGET /v1/calls/:id
ResponseStatus: 200 OK { "call": { "id": 812, "sid": "CA1234567890", "direct_link": "https://api.aircall.io/v1/calls/812", "direction": "outbound", "status": "done", "missed_call_reason": null, "started_at": 1584998199, "answered_at": 1584998205, "ended_at": 1584998210, "duration": 11, "voicemail": null, "recording": null, "asset": null, "raw_digits": "+1 800-123-4567", "user": { "id": 456, "direct_link": "https://api.aircall.io/v1/users/456", "name": "John Doe", "email": "john.doe@aircall.io", "available": true, "availability_status": "available", "created_at": "2019-12-29T10:03:18.000Z", "time_zone": "America/New_York", "language": "en-US" }, "contact": null, "archived": false, "assigned_to": null, "transferred_by": null, "transferred_to": null, "cost": "2.34", "number": { "id": 1234, "direct_link": "https://api.aircall.io/v1/numbers/1234", "name": "French Office", "digits": "+33 1 76 11 11 11", "created_at": "2020-01-02T11:41:01.000Z", "country": "FR", "time_zone": "Europe/Paris", "open": true, "availability_status": "custom", "is_ivr": true, "live_recording_activated": true, "priority": null, "messages": { ... } }, "comments": [ { "id": 735, "content": "Please call back this customer!", "posted_at": 1587994808, "posted_by": { "id": 456, "direct_link": "https://api.aircall.io/v1/users/456", "name": "Johnn Doe", "email": "john.doe@aircall.io", "available": true, "availability_status": "available", "created_at": "2019-12-29T10:03:18.000Z" } } ], "tags": [ { "id": 678, "name": "General Inquiries", "created_at": 1587995020, "tagged_by": { "id": 456, "direct_link": "https://api.aircall.io/v1/users/456", "name": "Johnn Doe", "email": "john.doe@aircall.io", "available": true, "availability_status": "available", "created_at": "2019-12-29T10:03:18.000Z" } }, ... ], "participants": [ { "name": "John Doe", "id": 456, "type": "user" }, { "name": "Jennifer Smith", "phone_number": "+33 7 49 88 29 71", "id": 5443, "type": "contact" }, { "name": "Rafael Lopez", "id": 457, "type": "user" } ], "teams": [], "ivr_options_selected": [ { "id": "5cb41cde-aed2-4357-a98c-e1b33d68851a", "title": "", "key": "1", "branch": "Default", "created_at": "2024-10-01T06:54:45.556Z", "transition_started_at": "2024-10-01T06:54:35.332Z", "transition_ended_at": "2024-10-01T06:54:45.513Z" } ] } }
Search for specific Calls depending on several Query Params like user_id
, phone_number
or tags
. Given a call transferred between A
and B
phone numbers, the call will not appear when filtering by A
but it will for B
.
Only three months of history is available. Please contact our Support team on support.aircall.io to get a one-time export of calls.
By default contact details are not added in response payload. To add the contact details in response set fetch_contact to
true
in query parameters.
-
Query params
-
fromStringSet a minimal creation date for Calls (UNIX timestamp).
-
toStringSet a maximal creation date for Calls (UNIX timestamp).
-
orderStringReorder entries by
created_at
. Can beasc
ordesc
.Default value isasc
. -
directionStringDirection of the Calls.If specified, can be
inbound
oroutbound
. -
user_idIntegerUnique ID of the User who made or received Calls.
-
phone_numberStringThe calling or receiving phone number of Calls.
-
tagsArrayArray of Tags IDs.Implemented as an
AND
condition: Aircall will search for Calls matching all the tags present in this array. -
fetch_contactBooleanWhen set to
true
, adds contacts details in response. -
fetch_short_urlsBooleanWhen set to
true
, adds short urls in response. -
fetch_call_timelineBooleanWhen set to
true
, it will return ivr_options_selected at the same level of the call
Using the pagination system, you can retrieve up to 10,000 Calls. To pass over this limit, we encourage you to use the
from
param as much as you can!
RequestGET /v1/calls/search
ResponseStatus: 200 OK { "meta": { "count": 3, "total": 2, "current_page": 1, "per_page": 20, "next_page_link": null, "previous_page_link": null }, "calls": [ { "id": 812, "sid": "CA1234567890", "direct_link": "https://api.aircall.io/v1/calls/812", "direction": "outbound", "status": "done", "missed_call_reason": null, "started_at": 1584998199, "answered_at": 1584998205, "ended_at": 1584998210, "duration": 11, "voicemail": null, "recording": null, "asset": null, "raw_digits": "+1 800-123-4567", "user": { "id": 456, "direct_link": "https://api.aircall.io/v1/users/456", "name": "John Doe", "email": "john.doe@aircall.io", "available": true, "availability_status": "available", "created_at": "2019-12-29T10:03:18.000Z", "time_zone": "America/New_York", "language": "en-US" }, "contact": null, "archived": false, "assigned_to": null, "transferred_by": null, "transferred_to": null, "cost": "2.34", "number": { "id": 1234, "direct_link": "https://api.aircall.io/v1/numbers/1234", "name": "French Office", "digits": "+33 1 76 11 11 11", "created_at": "2020-01-02T11:41:01.000Z", "country": "FR", "time_zone": "Europe/Paris", "open": true, "availability_status": "custom", "is_ivr": true, "live_recording_activated": true, "priority": null, "messages": { ... } }, "comments": [...], "tags": [...], "teams": [...], "ivr_options_selected": [...], }, { "id": 813, "direct_link": "https://api.aircall.io/v1/calls/813", "direction": "inbound", "status": "done", "missed_call_reason": null, "started_at": 1584998199, "answered_at": "no_available_agent", "ended_at": 1584998210, "duration": 22, "voicemail": null, "recording": null, "asset": null, "raw_digits": "+1 800-123-4567", "user": null, "contact": null, "archived": false, "assigned_to": null, "transferred_by": null, "transferred_to": null, "cost": "0", "number": { "id": 1234, "direct_link": "https://api.aircall.io/v1/numbers/1234", "name": "French Office", "digits": "+33 1 76 11 11 11", "created_at": "2020-01-02T11:41:01.000Z", "country": "FR", "time_zone": "Europe/Paris", "open": true, "availability_status": "custom", "is_ivr": true, "live_recording_activated": true, "priority": null, "messages": { ... } }, "comments": [...], "tags": [...], "teams": [...], "ivr_options_selected": [...], }, ... ] }
Calls can be transferred to another User, Team or phone number.
Transfers initiated through the API are only supported for scenarios where a call is transferred directly to a specific agent.
The transfer won’t reroute to other agents if the original transfer target is unavailable.
For more complex scenarios -such as those requiring multiple sequential transfers or fallback routing— we
recommend using the "Ring to via API" widget within Smartflows.
This widget allows for advanced routing logic and provides greater flexibility in managing call flows.
Only cold transfers are available via the Public API. Calls can be transferred to a single User, a single Team or a single external phone number:
- To transfer a Call to another User, provide a user ID in the request's body. A
call.transferred
Webhook event will be sent.
If the agent to whom the call was transferred to is not available, call will be directed to the No one answers strategy: the Unanswered call message will be played.
To transfer a Call to a Team, provide a team ID in the request's body. A
call.transferred
Webhook event will be sent. When transferring a call to a team dispatching strategy can be specified with parameter:dispatching_strategy
: The "simultaneous" strategy (default one if argument is not specified) will ring each available members simultaneously. The "random" one will ring each available member one by one. The "longest_idle" one will ring each available member one by one starting with the agent having the longest idle time.To transfer an Incoming Call to an external phone number, provide a phone number in the request body. When transfer is initiated, a message will be played to the caller informing them that their call is being transferred. A
call.transferred
Webhook event will be sent without the transferred_to field in the payload.
Transfers to external phone numbers will only work for inbound calls that have not yet been answered.
- If the transfer is not successful, a
call.unsuccessful_transfer
Webhook event will be sent.
-
Path params
-
idIntegerUnique identifier for the Call.
-
Body params
-
user_idStringUnique identifier of the User the Call will be transferred to.
-
team_idStringUnique identifier of the Team the Call will be transferred to.
-
numberStringExternal phone number the Call will be transferred to.Format must be e164. More information in the dedicated phone numbers format section.
-
dispatching_strategyStringSpecify dispatching strategy on team transfer, only values 'random', 'simultaneous' and 'longest_idle' are accepted. This parameter is optional if not specified 'simultaneous' strategy is implicitly used.
Only one of
user_id
, team_id
or number
parameters are allowed for each request.
code | description |
---|---|
204 | Success. |
400 | Call already ended. |
400 | Invalid usage of dispatching strategy with user or external phone number. |
400 | Multiple transfer destinies set on request. |
404 | User or Call not found. |
RequestPOST /v1/calls/:id/transfers { "user_id": 456 }
ResponseStatus: 204 No Content
Comments (also called Notes) can be added to Calls and a Call can have a maximum of five of them. After five comments added, those requests will fail with a 400
HTTP code. A comment posted via the Public API does not have an owner.
Once a Comment is posted on a Call, it cannot be updated nor deleted.
A
call.commented
Webhook event will be sent every time a call is commented. More info in the Webhooks section.
-
Path params
-
idIntegerUnique identifier for the Call.
-
Body params
-
contentStringContent of the Comment.Emojis are removed from Comments.
code | description |
---|---|
201 | Success. |
400 | Maximum of 5 notes can be added to a Call. |
RequestPOST /v1/calls/:id/comments { "content": "Please call back this customer!" }
ResponseStatus: 201 Created
Tags are created in the Dashboard by Admins and calls can be tagged by Agent from the Phone.
A
call.tagged
Webhook event will be sent every time a call is tagged. More info in the Webhooks section.
-
Path params
-
idIntegerUnique identifier for the Call.
-
Body params
-
tagsArrayArray of Tag IDs.
code | description |
---|---|
201 | Success. |
RequestPOST /v1/calls/:id/tags { "tags": [545] }
ResponseStatus: 201 Created
Missed calls non-archived will be displayed in the To-do view and can be Mark as done (= archived).
A
call.archived
Webhook event will be sent every time a call is archived. More info in the Webhooks section.
-
Path params
-
idIntegerUnique identifier for the Call.
RequestPUT /v1/calls/:id/archive
ResponseStatus: 200 OK { "call": { "id": 812, "sid": "CA1234567890", "direct_link": "https://api.aircall.io/v1/calls/812", "direction": "outbound", "status": "done", "missed_call_reason": null, "started_at": 1584998199, "answered_at": 1584998205, "ended_at": 1584998210, "duration": 11, "voicemail": null, "recording": null, "asset": null, "raw_digits": "+1 800-123-4567", "user": { "id": 456, "direct_link": "https://api.aircall.io/v1/users/456", "name": "John Doe", "email": "john.doe@aircall.io", "available": true, "availability_status": "available", "created_at": "2019-12-29T10:03:18.000Z", "time_zone": "America/New_York", "language": "en-US" }, "contact": null, "archived": true, "assigned_to": null, "transferred_by": null, "transferred_to": null, "cost": "2.34", "number": { "id": 1234, "direct_link": "https://api.aircall.io/v1/numbers/1234", "name": "French Office", "digits": "+33 1 76 11 11 11", "created_at": "2020-01-02T11:41:01.000Z", "country": "FR", "time_zone": "Europe/Paris", "open": true, "availability_status": "custom", "is_ivr": true, "live_recording_activated": true, "priority": null, "messages": { ... } }, "comments": [...], "tags": [...], "teams": [...] } }
Archived calls can be placed back in the To-do view of the Phone app.
-
Path params
-
idIntegerUnique identifier for the Call.
RequestPUT /v1/calls/:id/unarchive
ResponseStatus: 200 OK { "call": { "id": 812, "sid": "CA1234567890", "direct_link": "https://api.aircall.io/v1/calls/812", "direction": "outbound", "status": "done", "missed_call_reason": null, "started_at": 1584998199, "answered_at": 1584998205, "ended_at": 1584998210, "duration": 11, "voicemail": null, "recording": null, "asset": null, "raw_digits": "+1 800-123-4567", "user": { "id": 456, "direct_link": "https://api.aircall.io/v1/users/456", "name": "John Doe", "email": "john.doe@aircall.io", "available": true, "availability_status": "available", "created_at": "2019-12-29T10:03:18.000Z", "time_zone": "America/New_York", "language": "en-US", }, "contact": null, "archived": false, "assigned_to": null, "transferred_by": null, "transferred_to": null, "cost": "2.34", "number": { "id": 1234, "direct_link": "https://api.aircall.io/v1/numbers/1234", "name": "French Office", "digits": "+33 1 76 11 11 11", "created_at": "2020-01-02T11:41:01.000Z", "country": "FR", "time_zone": "Europe/Paris", "open": true, "availability_status": "custom", "is_ivr": true, "live_recording_activated": true, "priority": null, "messages": { ... } }, "comments": [...], "tags": [...], "teams": [...] } }
When live recording is actived on a Call, it can be automatically paused via the Public API.
-
Path params
-
idIntegerUnique identifier for the Call.
code | description |
---|---|
204 | Success. |
400 | Call has already ended. |
404 | Call not found. |
405 | Recording is disabled on the Call's number. |
RequestPOST /v1/calls/:id/pause_recording
ResponseStatus: 204 No Content
When live recording is actived on a Call and has been paused, it can be automatically resumed via the Public API.
-
Path params
-
idIntegerUnique identifier for the Call.
code | description |
---|---|
204 | Success. |
400 | Call has already ended. |
404 | Call not found. |
405 | Recording is disabled on the Call's Number. |
RequestPOST /v1/calls/:id/resume_recording
ResponseStatus: 204 No Content
Delete the recording of a specific Call. It takes between 10 and 15 minutes to delete a call recording from our servers.
Please note the recording can take up to 24 hours to be received. If it is not present, it will not be deleted. In this case retry the deletion later.
This action cannot be undone.
Check this endpoint to delete the voicemail of a Call.
-
Path params
-
idIntegerUnique identifier for the Call.
RequestDELETE /v1/calls/:id/recording
ResponseStatus: 200 OK
Delete the voicemail of a specific Call. It can take up to 1 minute to delete a call voicemail from our servers.
Please note the voicemail can take up to 24 hours to be received. If it is not present, it will not be deleted. In this case retry the deletion later.
This action cannot be undone.
Check this endpoint to delete the live recording of a Call.
-
Path params
-
idIntegerUnique identifier for the Call.
RequestDELETE /v1/calls/:id/voicemail
ResponseStatus: 200 OK
Insight Cards display custom data to Agents in their Phone apps during ongoing Calls.
Insight cards will only be seen on a ongoing calls and are not stored after Calls are over.
Three types of content can be sent in an Insight Card:
-
title
is a large text. It should describe the name of a custom app. -
shortText
is a normal line in the Insight Card section. -
user
is a user card in the Insight Card section displaying the name and availability of the user.
Please note Insight Card payload size should be less than 10KB.
More info on what Insight Cards are in our Knowledge Base.
-
Path params
-
idIntegerUnique identifier for the Call.
-
Body params
-
contentsArrayRepresents lines displayed on the Insight Card.Please check the following tables to see how to structure this object.
-
Title
-
typeStringType of line. Should be
title
.Mandatory field. Possible values aretitle
,shortText
oruser
(check other tables). -
textStringText displayed in the line of the Insight Card.Mandatory field.
-
linkStringTransform the line in a link.
-
Short text
-
typeStringType of line. Should be
shortText
.Mandatory field. Possible values aretitle
,shortText
oruser
(check other tables). -
textStringText displayed in the title field of the Insight Card.Mandatory field.
-
linkStringTransform the line in a link.
-
labelStringThe
text
field can be preceded by alabel
if one is provided.
-
User
-
typeStringType of line. Should be
user
.Mandatory field. Possible values aretitle
,shortText
oruser
(check other tables). -
labelStringThe text that preceeds the user card.Mandatory field.
-
user_idIntegerThe id of the user to be displayed.Mandatory field.
code | description |
---|---|
201 | Success. |
400 | Payload malformed or size greater than 10KB. |
404 | Call not found. |
RequestPOST /v1/calls/:id/insight_cards { "contents": [ { "type": "title", "text": "My Custom CRM", "link": "https://my-custom-crm.com" }, { "type": "shortText", "label": "Account ID", "text": "6789", "link": "https://my-custom-crm.com/6789" }, { "type": "shortText", "label": "Company name", "text": "Acme Inc." }, { "type": "user", "label": "Account Owner", "user_id": 12345 } ] }
ResponseStatus: 201 Created
Dialer Campaign
Dialer Campaigns refer to the Power Dialer feature. More info on how it works in our Knowledge Base.
Dialer Campaigns are composed of one or several phone numbers.
-
Attributes
-
idIntegerUnique identifier for the Dialer Campaign.
-
number_idStringSet if the Dialer Campaign is associated to a Number.
-
created_atStringDialer campaign's creation date, in UTC.
-
Phone number attributes
-
idIntegerUnique identifier for the phone number.
-
numberStringThe actual phone number.
-
calledBoolean
true
if the number was called by the User. -
created_atStringPhone number's creation date, in UTC.
EndpointsGET /v1/users/:user_id/dialer_campaignRetrieve a Dialer Campaign POST /v1/users/:user_id/dialer_campaignCreate a Dialer Campaign DELETE /v1/users/:user_id/dialer_campaignDelete a Dialer Campaign GET /v1/users/:user_id/dialer_campaign/phone_numbersRetrieve phone numbers POST /v1/users/:user_id/dialer_campaign/phone_numbersAdd phone numbers DELETE /v1/users/:user_id/dialer_campaign/phone_numbers/:idDelete a phone number
A User can have only one active Dialer Campaign.
To list all the phone numbers associated to it, please refer to the Retrieve phone numbers endpoint.
-
Path params
-
user_idIntegerUnique identifier for the User.
code | description |
---|---|
404 | User has no active campaign. |
RequestGET /v1/users/:user_id/dialer_campaign
ResponseStatus: 200 OK { "id": 75555, "number_id": null, "created_at": "2020-03-21T20:20:04.000Z" }
Create an active Dialer Campaign for a User.
A User can have only one active Dialer Campaign.
-
Path params
-
user_idIntegerUnique identifier for the User.
-
Body params
-
phone_numbersArrayArray of valid phone numbers.Mandatory field. Must include at least one valid phone number.
code | description |
---|---|
422 | Must include at least one valid phone number. |
RequestPOST /v1/users/:user_id/dialer_campaign { "phone_numbers": ["+19161112222", "+18001112222"] }
ResponseStatus: 204 No Content
Dialer Campaign created by Agents or via the Public API can be deleted.
Phone numbers associated to it will be destroyed as well.
-
Path params
-
user_idIntegerUnique identifier for the User.
code | description |
---|---|
404 | User has no active campaign. |
RequestDELETE /v1/users/:user_id/dialer_campaign
ResponseStatus: 204 No Content
A Dialer Campaign is made of a list of phone numbers. Those lists can be retrieved thanks to the following request.
-
Path params
-
user_idIntegerUnique identifier for the User.
code | description |
---|---|
404 | User has no active campaign. |
RequestGET /v1/users/:user_id/dialer_campaign/phone_numbers
ResponseStatus: 200 OK { "numbers": [ { "id": 4671488, "number": "+18002112223", "called": false, "created_at": "2020-03-20T20:42:57.000Z" }, { "id": 4671489, "number": "+18003112224", "called": false, "created_at": "2020-03-20T20:42:57.000Z" }, { "id": 4671490, "number": "+18004112225", "called": false, "created_at": "2020-03-20T20:42:57.000Z" } ] }
Phone numbers can be added to an Agent's active Dialer Campaign.
Those phone numbers will be automatically appended in the Phone app.
-
Path params
-
user_idIntegerUnique identifier for the User.
-
Body params
-
phone_numbersArrayArray of valid phone numbers.Mandatory field. Must include at least one valid phone number.
code | description |
---|---|
422 | Must include at least one valid phone number. |
RequestPOST /v1/users/:user_id/dialer_campaign/phone_numbers { "phone_numbers": [ "+19161112222", "+18001112222" ] }
ResponseStatus: 204 No Content
Delete one phone number from a Dialer Campaign.
-
Path params
-
user_idIntegerUnique identifier for the User.
-
idIntegerUnique identifier for the phone number.
RequestDELETE /v1/users/:user_id/dialer_campaign/phone_numbers/:id
ResponseStatus: 204 No Content
Number
Users (Admins) can buy Numbers from the Dashboard. They can then update the call distribution order, upload custom Music & Messages, enable or disable call recording...
A Number can be either Classic or IVR:
- Classic: Assigned users and teams will make and receive calls from that number.
- IVR (Interactive Voice Response): Callers will reach a voice menu first, and choose among options through their keypad.
-
Attributes
-
idIntegerUnique identifier for the Number.
-
direct_linkStringDirect API URL.
-
nameStringThe name of the Number.
-
digitsStringInternational format of the Number.
-
e164_digitsStringNumber in E.164 formatNumber in following format [+][country code][area code][local phone number] i.e. without any space or special characters e.g. +18001234567, +33176360695. Only available in Webhook events
-
created_atStringTimestamp when the Number was created, in UTC.
-
countryStringISO 3166-1 alpha-2 country code of the Number.Listed on Wikipedia.
-
time_zoneStringNumber's time zone, set in the Dashboard.More details on Timezones here.
-
openBooleanCurrent opening state of the Number, based on its opening hours.
-
availability_statusStringCurrent availability status of the Number.
open
,custom
,closed
-
is_ivrBoolean
true
if Number is an IVR,false
if Number is a Classic Number.More information on our Knowledge Base. -
live_recording_activatedBooleanWhether a Number has live recording activated or not.More information on our Knowledge Base.
-
usersArrayList of Users linked to this Number.
-
priorityIntegerPriority level of the number used during routing of the callsCan be
null
,0
(no priority) or1
(top priority). Default value isnull
-
messagesObjectURL to Number's music & messages files.See Music & Messages section below for more details.
Music and Messages
The messages
object, present for each Number, represents the different audio files played during a call.
A custom file can be used for any of the following field by uploading it on a server with a public URL to it. Check our encoding recommendations on our Knowledge Base first!
-
Attributes
-
welcomeStringWelcome message URL. This file is played at the beginning of an incoming call.Available for Classic & IVR numbers.
-
waitingStringWaiting music URL. Caller will hear this if they are put on hold during an ongoing call or while the call is being transfered.Available for Classic & IVR numbers.
-
ringing_toneStringRinging tone URL. During an incoming call, caller will hear this music while waiting for the call to be answered.Available for Classic numbers.
-
unanswered_callStringUnanswered Call message URL. Caller will hear this message if their call is not answered when the business hours are open.Available for Classic numbers.
-
after_hoursStringAfter Hours message URL. Caller will hear this message if they call outside of this number's business hours.Available for Classic numbers.
-
ivrStringIVR message URL. Caller will hear this right after the Welcome message. This message will be played twice.Available for IVR numbers.
-
voicemailStringVoicemail message URL. Deprecated: replaced by
unanswered_call
.Available for IVR numbers. -
closedStringClosed message URL. Deprecated: replaced by
after_hours
.Available for IVR numbers. -
callback_laterStringCallback Later message.Available for IVR numbers.
Fetch all Numbers associated to a company and their information.
-
Query params
-
fromStringSet a minimal creation date for Numbers (UNIX timestamp).
-
toStringSet a maximal creation date for Numbers (UNIX timestamp).
-
orderStringReorder entries by
created_at
. Can beasc
ordesc
.Default value isasc
Pagination params can be used on this request.
RequestGET /v1/numbers
ResponseStatus: 200 OK { "meta": { "count": 3, "total": 2, "current_page": 1, "per_page": 20, "next_page_link": null, "previous_page_link": null }, "numbers": [ { "id": 1234, "direct_link": "https://api.aircall.io/v1/numbers/1234", "name": "French Office", "digits": "+33 1 76 11 11 11", "created_at": "2020-01-02T11:41:01.000Z", "country": "FR", "time_zone": "Europe/Paris", "open": true, "availability_status": "custom", "is_ivr": true, "live_recording_activated": true, "priority": null, "messages": { "welcome": "https://example.com/welcome.mp3", "waiting": "https://example.com/waiting_music.mp3", "ivr": "https://example.com/ivr_message.mp3", "voicemail": "https://example.com/voicemail.mp3", "closed": "https://example.com/closed_message.mp3", "callback_later": "https://example.com/callback_later.mp3", "unanswered_call": "https://example.com/unanswered_call.mp3", "after_hours": "https://example.com/closed_message.mp3", "ringing_tone": "https://example.com/waiting_music.mp3" } }, { "id": 2345, "direct_link": "https://api.aircall.io/v1/numbers/2345", "name": "UK office", "digits": "+44 20 0000 0000", "created_at": "2020-01-04T19:28:58.000Z", "country": "GB", "time_zone": "Europe/London", "open": true, "availability_status": "custom", "is_ivr": true, "live_recording_activated": false, "priority": null, "messages": { "welcome": "https://example.com/welcome.mp3", "waiting": "https://example.com/waiting_music.mp3", "ivr": "https://example.com/ivr_message.mp3", "voicemail": "https://example.com/voicemail.mp3", "closed": "https://example.com/closed_message.mp3", "callback_later": "https://example.com/callback_later.mp3", "unanswered_call": "https://example.com/unanswered_call.mp3", "after_hours": "https://example.com/closed_message.mp3", "ringing_tone": "https://example.com/waiting_music.mp3" } } ] }
Retrieve details of a specific Numbers.
-
Path params
-
idIntegerUnique identifier for the Number.
RequestGET /v1/numbers/:id
ResponseStatus: 200 OK { "number": { "id": 1234, "direct_link": "https://api.aircall.io/v1/numbers/1234", "name": "French Office", "digits": "+33 1 76 11 11 11", "created_at": "2020-01-02T11:41:01.000Z", "country": "FR", "time_zone": "Europe/Paris", "open": true, "availability_status": "custom", "is_ivr": true, "live_recording_activated": true, "priority": null, "messages": { "welcome": "https://example.com/welcome.mp3", "waiting": "https://example.com/waiting_music.mp3", "ivr": "https://example.com/ivr_message.mp3", "voicemail": "https://example.com/voicemail.mp3", "closed": "https://example.com/closed_message.mp3", "callback_later": "https://example.com/callback_later.mp3", "unanswered_call": "https://example.com/unanswered_call.mp3", "after_hours": "https://example.com/closed_message.mp3", "ringing_tone": "https://example.com/waiting_music.mp3" }, "users": [ { "id": 456, "direct_link": "https://api.aircall.io/v1/users/456", "name": "John Doe", "email": "john.doe@aircall.io", "available": true, "availability_status": "available", "created_at": "2019-12-29T10:03:18.000Z", "time_zone": "America/New_York" } ] } }
Updates a specific Number. Response will be the updated Number.
-
Path params
-
idIntegerUnique identifier for the Number.
-
Body params
-
nameStringCan't be empty or
null
. -
availability_statusStringCan be
open
,custom
orclosed
.This setting has been discontinued for Smartflows numbers. The concept of Business Hours (in Number settings) has been removed and replaced with the Time Rule widget in the Smartflows Editor. To handle holiday closures or other date-specific updates, we recommend using the Date Rule widget. Admins can configure date-specific routing by adding a Date Rule widget at the top of the flow. -
is_ivrBooleanIf
true
, Number will be converted into an IVR. Classic otherwise.This setting has been discontinued as the distinction between Classic and IVR numbers is no longer needed. IVR functionality can now be configured directly within the Smartflows Editor for any number. -
live_recording_activatedBooleanSet to
true
if you want to enable call recording on the specified Number. It will enable both inbound AND outbound at the same time.More information on our Knowledge Base. -
priorityIntegerCan be
null
,0
(no priority) or1
(top priority). Default value isnull
-
messagesObjectSee Update a Number's Music & Messages section.It is not yet possible to update messages for Smartflows numbers via the Public API. We are actively working to enable this feature.
RequestPUT /v1/numbers/:id { "name": "French Office UPDATED" }
ResponseStatus: 200 OK { "number": { "id": 1234, "direct_link": "https://api.aircall.io/v1/numbers/1234", "name": "French Office UPDATED", "digits": "+33 1 76 11 11 11", "created_at": "2020-01-02T11:41:01.000Z", "country": "FR", "time_zone": "Europe/Paris", "open": true, "availability_status": "custom", "is_ivr": true, "live_recording_activated": true, "priority": null, "messages": { "welcome": "https://example.com/welcome.mp3", "waiting": "https://example.com/waiting_music.mp3", "ivr": "https://example.com/ivr_message.mp3", "voicemail": "https://example.com/voicemail.mp3", "closed": "https://example.com/closed_message.mp3", "callback_later": "https://example.com/callback_later.mp3", "unanswered_call": "https://example.com/unanswered_call.mp3", "after_hours": "https://example.com/closed_message.mp3", "ringing_tone": "https://example.com/waiting_music.mp3" }, "users": [ { "id": 3456, "direct_link": "https://api.aircall.io/v1/users/3456", "created_at": "2019-12-29T10:03:18.000Z", "name": "John Doe", "email": "john.doe@aircall.io", "available": true, "availability_status": "available", "time_zone": "America/New_York" } ] } }
A Number's Music & Messages URLs can be updated by using the same request as to update a Number. Response will be the targeted Number, with updated messages
section.
It is not possible to perform automatic updates to music and messages for Smartflows numbers via the Public API. We are actively working to enable this functionality by the End of Life of Legacy Routing. More information can be found here.
-
Query params
-
idIntegerUnique identifier for the Number.
-
Body params
-
messagesObjectCheck the
messages
object format below.
-
Messages object
-
welcomeStringMust be a valid public URL.
-
waitingStringMust be a valid public URL.
-
ivrStringMust be a valid public URL.
-
voicemailStringMust be a valid public URL.
-
closedStringMust be a valid public URL.
-
callback_laterStringMust be a valid public URL.
-
unanswered_callStringMust be a valid public URL.
-
after_hoursStringMust be a valid public URL.
-
ringing_toneStringMust be a valid public URL.
For more information on each
messages
field, please refer to the Number object overview.
RequestPUT /v1/numbers/:id { "messages": { "welcome": "https://example.com/welcome_NEW.mp3" } }
ResponseStatus: 200 OK { "number": { "id": 1234, "direct_link": "https://api.aircall.io/v1/numbers/1234", "name": "French Office UPDATED", "digits": "+33 1 76 11 11 11", "created_at": "2020-01-02T11:41:01.000Z", "country": "FR", "time_zone": "Europe/Paris", "open": true, "availability_status": "custom", "is_ivr": true, "live_recording_activated": true, "priority": null, "messages": { "welcome": "https://example.com/welcome.mp3", "waiting": "https://example.com/waiting_music.mp3", "ivr": "https://example.com/ivr_message.mp3", "voicemail": "https://example.com/voicemail.mp3", "closed": "https://example.com/closed_message.mp3", "callback_later": "https://example.com/callback_later.mp3", "unanswered_call": "https://example.com/unanswered_call.mp3", "after_hours": "https://example.com/closed_message.mp3", "ringing_tone": "https://example.com/waiting_music.mp3" }, "users": [ ... ] } }
Fetch compliance status for :
- A2P10DLC messaging registration in the US
- Toll-free messaging registration in the US & Canada
for a specific Numbers.
-
Path params
-
idIntegerUnique identifier for the Number.
-
Headers
-
content-typestringContent type of the request. It will be application/json.
Send message function & number configuration for such use case can be found under “Message” section here.
RequestGET /v1/numbers/:id/registration_status
ResponseStatus: 200 OK { "status": "approved" "updatedAt": "2024-05-07T10:42:55.676Z", }
Conversation Intelligence
The Conversation Intelligence object is a representation of different AI-generated entities such as Key Topics, Call Transcription, Sentiment Analysis etc.
Conversation Intelligence object is not updatable nor destroyable via Aircall Public API.
The Conversation Intelligence object and APIs can only be retrieved if a company has signed up for Aircall AI Package
-
Attributes
-
idIntegerUnique identifier for the Conversation Intelligence event.
-
call_idStringUnique identifier for the call.For sentiment.created event
id
andcall_id
will have same value -
call_uuidStringUnique identifier for the call.Available via Webhook events only
-
number_idIntegerUnique identifier for the number.This field is only applicable for transcription.created event
-
participantsArrayParticipants involved in the call.
-
typestringType of the call. It will be 'call' or 'voicemail'.This field is only applicable for transcription.created event and to retrieve a transcription
-
contentstring or Array or ObjectThe Content of the Conversation Intelligence objectThis field is applicable for retrieve a transcription
This field is applicable for retrieve topics
This field for retrieve a summary -
call_created_atStringTimestamp when the call was created, in UTC.This field is applicable for retrieve a transcription
-
created_atStringTimestamp when the topics were created, in UTC.
Use this endpoint to retrieve a transcription.
To get transcription for a call in real time, we recommend you to subscribe to transcription.created event to get intimated about availability of Transcription for a call. This can be useful if you want to get intimation about when transcription is generated and will like to use API to retrieve and store it. Also, please note Transcription API doesn't contains any info related to the Calls. Please use Calls APIs to retrieve call details.
-
Path params
-
call_idIntegerUnique identifier for the Call.
code | description |
---|---|
200 | Success. |
400 | Validation error: invalid call_id. |
403 | Forbidden access: company is not active. |
403 | Forbidden access: company is not verified. |
500 | Internal server error: unknown error. |
RequestGET /v1/calls/:call_id/transcription
ResponseStatus: 200 OK { "transcription": { "id": 68271, "call_id": 5237603, "call_created_at": "2024-07-26T13:30:54.000Z", "type": "call", "content": { "language": "en", "utterances": [ { "start_time": 12.54, "end_time": 13.8, "text": "Okay,", "participant_type": "external", "phone_number": "+33679198915" }, { "start_time": 238.08, "end_time": 239.48, "text": "Okay, I guess that's enough.", "participant_type": "internal", "user_id": 123 } ] } } }
Use this endpoint to retrieve sentiments.
-
Path params
-
call_idIntegerUnique identifier for the Call.
code | description |
---|---|
200 | Success. |
400 | Validation error: invalid call_id. |
403 | Forbidden access: company is not active. |
403 | Forbidden access: company is not verified. |
500 | Internal server error: unknown error. |
RequestGET /v1/calls/:call_id/sentiments
ResponseStatus: 200 OK { "sentiment": { "id": 5237603, "call_id": 5237603, "participants": [ { "phone_number": "+33679198915", "value": "POSITIVE", "type": "external" } ] } }
Use this endpoint to retrieve topics from a call.
-
Path params
-
call_idIntegerUnique identifier for the Call.
code | description |
---|---|
200 | Success. |
400 | Validation error: invalid call_id. |
403 | Forbidden access: company is not active. |
403 | Forbidden access: company is not verified. |
500 | Internal server error: unknown error. |
RequestGET /v1/calls/:call_id/topics
ResponseStatus: 200 OK { "topic": { "id": 786, "call_id": 123, "created_at": "2024-08-27T10:54:16.000Z", "content": [ "payment", "billing" ] } }
Use this endpoint to retrieve summary from a call.
-
Path params
-
call_idIntegerUnique identifier for the Call.
code | description |
---|---|
200 | Success. |
400 | Validation error: invalid call_id. |
403 | Forbidden access: company is not active. |
403 | Forbidden access: company is not verified. |
500 | Internal server error: unknown error. |
RequestGET /v1/calls/:call_id/summary
ResponseStatus: 200 OK { "summary": { "id": 974, "call_id": 786, "created_at": "2024-08-27T10:54:16.000Z", "content": "Short summary of the call" } }
Content
The Content object is a representation of the content of Conversation Intelligence
Content are not updatable nor destroyable via Aircall Public API.
Content information is only present in transcriptions, summaries, topics and it can be accessed inside the conversation intelligence object
Please note the attributes of Content Object are different for each type of Conversation Intelligence.
Attributes list for Transcription Object
-
Attributes
-
contentObjectThe content of the transcription
-
languageStringThe language of the transcription. Possible values:
en
,en-US
,en-GB
,en-AU
,fr-FR
,fr
,es-ES
,es
,de-DE
,de
,nl-NL
,nl
,it-IT
,it
-
utterancesArraySentences of the transcription
-
utterances.start_timeNumberTime when the sentence started
-
utterances.end_timeNumberTime when the sentence ended
-
utterances.textStringThe sentence content
-
utterances.participant_typeStringThe type of participant
external
orinternal
-
utterances.user_idNumberThe user_id when it's an
internal
participant -
utterances.phone_numberStringThe phone number when it's an
external
participant
Attributes list for Summary Object
-
Attributes
-
contentStringThe summary content
Attributes list for Topics Object
-
Attributes
-
contentArrayAn array of string with all topics of the call
Message
Messages are an essential part of how Aircall users interact with the product. Our mission is to make the communication experience as delightful as possible!
There are two types of messages:
- Inbound Messages: when a user receives a message from an external person.
- Outbound Messages: when a user sends a message to an external person.
Message objects are neither updatable nor destroyable via Aircall Public API.
-
Attributes
-
idStringA unique identifier for the message.
-
direct_linkStringDirect API URL.
-
directionStringDirection of the Message.Could be inbound or outbound.
-
external_numberStringThe phone number associated with the message, including the country code.
-
bodyStringThe content or text of the message.
-
statusStringThe status of the message.
-
raw_digitsStringInternational format of the number.
-
media_detailsStringA list of media files attached to the message.for each media_details, file_name
string
, file_typestring
and presigned_urlstring
fields are also required. -
created_atStringTimestamp when the Message was created, in UTC.
-
updated_atStringTimestamp when the Message was updated for the last time, in UTC.
-
sent_atStringTimestamp when the Message was sent, in UTC.
-
numberObjectFull Number object attached to the Message.
-
contactObjectFull Contact object attached to the Message.
EndpointsPOST /v1/numbers/:id/messages/configurationCreate Number Configuration GET /v1/numbers/:id/messages/configurationFetch Number Configuration DELETE /v1/numbers/:id/messages/configurationDelete Number Configuration POST /v1/numbers/:id/messages/sendSend Message POST /v1/numbers/:id/messages/native/sendSend Message in agent conversation
Create or update the number configuration to allow number to use the Send message endpoint.
Once numbers are configured, they won't be able to send or receive messages via aircall apps.
Numbers meant to be used with Send message in agent conversation do not require to be configured.
For US long codes and US/CA toll free lines, numbers must be registered before configuration. See here to check registration status
Set a callbackURL that will be used not only to retrieve the status of messages sent, but also to get the messages received. Webhooks to retrieve messages activity do not expose messages sent and received with numbers that are active using the configuration exposed in this section
-
Path params
-
idIntegerUnique identifier for the Number.
-
Body params
-
callbackUrlStringThe callback url will be used to retrieve status update of the message sent, and possible answers from the recipient.Optional field
-
removeExistingConfigBooleanIf there is any existing configuration with a third party messaging application from the line then it will be removed.Optional field
code | description |
---|---|
200 | Success. |
400 | Validation error: the line is not covered. |
400 | Validation error: IVR lines are not supported. |
400 | Validation error: line is not SMS capable. |
403 | Forbidden access: line does not belong to the company. |
403 | Forbidden access: company is not active. |
403 | Forbidden access: company is not verified. |
403 | Forbidden access: line is not configured to use public api. |
RequestPOST /v1/numbers/:id/messages/configuration { "callbackUrl":"yourCallbackUrl" "removeExistingConfig": false }
ResponseStatus: 200 OK { "token": "token", "callbackUrl": "yourCallbackUrl", "type": "PublicApi", }
Fetch settings associated to a number Numbers and their information for Aircall Messaging public api.
-
Query params
-
idIntegerUnique identifier for the Number.
-
Headers
-
content-typestringContent type of the request. It will be application/json.
code | description |
---|---|
200 | Success. |
403 | Forbidden access: line does not belong to the company. |
404 | Validation Error: Line not configured for Public Api. |
RequestGET /v1/numbers/:id/messages/configuration
ResponseStatus: 200 OK { "token": "token", "callbackUrl": "yourCallbackUrl", "type": "PublicApi", }
Delete configuration associated to a number Numbers for Aircall Messaging public api.
-
Query params
-
idIntegerUnique identifier for the Number.
code | description |
---|---|
204 | Success. |
403 | Forbidden access: line does not belong to the company. |
404 | Validation Error: Line not configured for Public Api. |
RequestDELETE /v1/numbers/:id/messages/configuration
ResponseStatus: 204 No Content
Send a text message or a multimedia message from a public api configured number.
Numbers not configured won't be able to send messages via API. Number can be configured from here.
-
Query params
-
idIntegerUnique identifier for the Number.
-
Body params
-
toStringnumber you want to send the messageMandatory field
-
bodyStringtext you want to send
-
mediaUrlArray<String>media urls you want to sendOptional field
code | description |
---|---|
200 | Success. |
400 | Validation error: the line is not covered. |
400 | Validation error: the carrier line is not supported. |
400 | Validation error: IVR lines are not supported. |
400 | Validation error: line is not SMS capable. |
400 | Validation error: the phone number is not sms capable. |
400 | Validation error: the conversation between this line and this external number is not allowed. |
400 | Validation error: the message does not have content. |
400 | Validation error: message length exceeds the maximum of 1600 characters. |
400 | Validation error: the receiver should be different from the sender. |
403 | Forbidden access: messaging public api is not enabled for this company. |
403 | Forbidden access: line does not belong to the company. |
403 | Forbidden access: public api sms is not enabled for this line. |
403 | Forbidden Exception: the line exceeded number of messages limit. |
403 | Forbidden Exception: the line exceeded number of message limit for company on trial. |
403 | Forbidden access: company is not active. |
403 | Forbidden access: company is not verified. |
500 | Outbound message xxxx not sent. |
RequestPOST /v1/numbers/:id/messages/send { "to": "+130...", "body": "text you want to send", "mediaUrl": ["", ""] }
ResponseStatus: 200 OK { "id": "messageId", "status": "pending", "direct_link": "https://api.aircall.io/v1/numbers/:lineId/messages/:messageId", "direction": "outbound", "created_at": 1722317361216, "sent_at": 1722317361216, "updated_at": 1722317361216, "raw_digits": "+130...", "media_url": ["", ""], "body": "text you want to send", ...number object }
Send a text message from an aircall SMS-capable number that will be stored and added in the agent conversation.
Any agent added to the number will be able to see the message in their aircall apps and will be able to continue the conversation with the recipient. If no conversation with the recipient already exists, it will be created then.
Any number configured to be used for Send message endpoint won’t be able to use this endpoint. Configuration needs to be deleted first.
This endpoint is not meant for high volume use cases or non conversational use cases, for such use cases use this endpoint. Too many messages sent via this endpoint will disrupt agents work within the aircall apps.
-
Query params
-
idIntegerUnique identifier for the Number.
-
Body params
-
toStringnumber you want to send the messageMandatory field
-
bodyStringtext you want to sendMandatory field
code | description |
---|---|
200 | Success. |
400 | Validation error: the line is not covered. |
400 | Validation error: the carrier line is not supported. |
400 | Validation error: IVR lines are not supported. |
400 | Validation error: line is not SMS capable. |
400 | Validation error: the phone number is not sms capable. |
400 | Validation error: the conversation between this line and this external number is not allowed. |
400 | Validation error: the message does not have content. |
400 | Validation error: message length exceeds the maximum of 1600 characters. |
400 | Validation error: the receiver should be different from the sender. |
403 | Forbidden access: messaging public api is not enabled for this company. |
403 | Forbidden access: line does not belong to the company. |
403 | Forbidden access: public api sms is not enabled for this line. |
403 | Forbidden Exception: the line exceeded number of messages limit. |
403 | Forbidden Exception: the line exceeded number of message limit for company on trial. |
403 | Forbidden access: company is not active. |
403 | Forbidden access: company is not verified. |
500 | Outbound message xxxx not sent. |
RequestPOST /v1/numbers/:id/messages/native/send { "to": "+130...", "body": "text you want to send", }
ResponseStatus: 200 OK { "id": "messageId", "status": "pending", "direct_link": "https://api.aircall.io/v1/numbers/:lineId/messages/:messageId", "direction": "outbound", "created_at": 1722317361216, "sent_at": 1722317361216, "updated_at": 1722317361216, "raw_digits": "+130...", "media_url": ["", ""], "body": "text you want to send", ...number object, }
Contact
Contacts are a core part of Aircall experience.
Every time Agents are on a call, Aircall fetches their contact information from different sources.
Please note that contacts created by third parties integrations will not be accessible from the public-api, but you will still be able to see them in the phone app (mobile & desktop)
To better understand how contacts sync from third-party integrations works, you can have a look at this article from our knowledge base.
Contacts CSV files can be uploaded by Agents in the Phone app.
More info here.
-
Attributes
-
idIntegerUnique identifier for the Contact.
-
direct_linkStringDirect API URL.
-
first_nameStringContact's first name.
-
last_nameStringContact's last name.
-
company_nameStringContact's company name.
-
descriptionStringField used by Aircall to qualify tags.
-
informationStringExtra information about the contact.Can be used to store outside data.
-
is_sharedBooleanContact can be shared within the organization.All Contacts retrieved via the Public API are shared.
-
phone_numbersArrayPhone numbers of this contact.More details in the Phone numbers attribute table below.
-
emailsArrayEmail addresses of this contact.More details in the Emails attribute table below.
-
Phone numbers attribute
-
idIntegerUnique identifier for this phone number.
-
labelStringA custom label like
work
,home
... -
valueStringThe raw phone number.The value needs to be valid
-
Emails attribute
-
idIntegerUnique identifier for this email address.
-
labelStringA custom label like
work
,home
... -
valueStringThe email address.
Emojis can't be used in Contact's attributes (they will be removed).
EndpointsGET /v1/contactsList all Contacts GET /v1/contacts/searchSearch Contacts GET /v1/contacts/:idRetrieve a Contact POST /v1/contactsCreate a Contact POST /v1/contacts/:idUpdate a Contact DELETE /v1/contacts/:idDelete a Contact POST /v1/contacts/:id/phone_detailsAdd phone number to a Contact PUT /v1/contacts/:id/phone_details/:phone_number_idUpdate a phone number from a Contact DELETE /v1/contacts/:id/phone_details/:phone_number_idDelete a phone number from a Contact POST /v1/contacts/:id/email_detailsAdd email to a Contact PUT /v1/contacts/:id/email_details/:email_idUpdate an email from a Contact DELETE /v1/contacts/:id/email_details/:email_idDelete an email from a Contact
Fetch all the shared Contacts associated to a company with their phone numbers and emails information.
-
Query params
-
fromStringSet a minimal creation date for contacts (UNIX timestamp).
-
toStringSet a maximal creation date for contacts (UNIX timestamp).
-
orderStringReorder entries by order_by,
created_at
otherwise. Can beasc
ordesc
.Default value isasc
-
order_byStringSet the order field,
created_at
orupdated_at
.Default value iscreated_at
Using the pagination system, you can retrieve up to 10,000 Contacts. To pass over this limit, we encourage you to use the
from
param as much as you can!
RequestGET /v1/contacts
ResponseStatus: 200 OK { "meta": { "count": 2, "total": 2, "current_page": 1, "per_page": 20, "next_page_link": null, "previous_page_link": null }, "contacts": [ { "id": 710, "direct_link": "https://api.aircall.io/v1/contacts/710", "first_name": "Vicente", "last_name": "Abad", "company_name": null, "information": null, "is_shared": true, "created_at": 1400691054, "updated_at": 1444336506, "emails": [], "phone_numbers": [ { "id": 3367, "label": "Mobile", "value": "+34664673697" } ] }, { "id": 711, "direct_link": "https://api.aircall.io/v1/contacts/711", "first_name": "Margaret", "last_name": "Morrison", "company_name": "TeleWorm", "information": null, "is_shared": true, "created_at": 1400692007, "updated_at": 1444336507, "emails": [], "phone_numbers": [ { "id": 3368, "label": "Mobile", "value": "+34768776683" }, { "id": 3369, "label": "Work", "value": "+49111000460" } ], "emails": [ { "id": 3200, "label": "Work", "value": "m.morrison@teleworm.es" } ] } ] }
Search within a company's shared Contacts with extra query parameters.
-
Query params
-
fromStringSet a minimal creation date for contacts (UNIX timestamp).
-
toStringSet a maximal creation date for contacts (UNIX timestamp).
-
orderStringReorder entries by order_by,
created_at
otherwise. Can beasc
ordesc
.Default value isasc
-
order_byStringSet the order field,
created_at
orupdated_at
.Default value iscreated_at
-
phone_numberStringPhone number of a contact
-
emailStringEmail address of a contact
Using the pagination system, you can retrieve up to 10,000 Contacts. To pass over this limit, we encourage you to use the
from
param as much as you can!
RequestGET /v1/contacts/search
ResponseStatus: 200 OK { "meta": { "count": 1, "total": 1, "current_page": 1, "per_page": 20, "next_page_link": null, "previous_page_link": null }, "contacts": [ { "id": 711, "direct_link": "https://api.aircall.io/v1/contacts/711", "first_name": "Margaret", "last_name": "Morrison", "company_name": "TeleWorm", "information": null, "is_shared": true, "created_at": 1400692007, "updated_at": 1444336507, "emails": [], "phone_numbers": [ { "id": 3368, "label": "Mobile", "value": "+34768776683" }, { "id": 3369, "label": "Work", "value": "+49111000460" } ], "emails": [ { "id": 3200, "label": "Work", "value": "m.morrison@teleworm.es" } ] } ] }
Retrieve details of a specific Contact.
Only shared contacts can be found.
-
Path params
-
idIntegerUnique identifier for the Contact.
RequestGET /v1/contacts/:id
ResponseStatus: 200 OK { "contact": { "id": 710, "direct_link": "https://api.aircall.io/v1/contacts/710", "first_name": "Vicente", "last_name": "Abad", "company_name": null, "information": null, "is_shared": true, "created_at": 1400691054, "updated_at": 1444336506, "emails": [], "phone_numbers": [ { "id": 3367, "label": "Mobile", "value": "+34664673697" } ] } }
Contacts created via Aircall Public API will be automatically shared across the company.
phone_numbers
is required when creating a Contact.
-
Body params
-
first_nameStringCan't be blank if defined.Can’t exceed 255 characters
-
last_nameStringCan't be blank if defined.Can’t exceed 255 characters
-
company_nameStringCan't be blank if defined.Can’t exceed 255 characters
-
informationStringCan't be blank if defined.
-
emailsArrayArray of email address objects.For each email,
label
andvalue
must be set. Limit of 20 emails, beyond, a 400 error will be returned -
phone_numbersArrayArray of phone_numbers objects.Mandatory field. For each phone_number,
label
andvalue
must be set. Limit of 20 phone numbers, beyond, a 400 error will be returned
Duplicate calls to POST /v1/contacts with the same payload will create duplicate contacts.
RequestPOST /v1/contacts { "first_name": "Gary", "last_name": "Jennings", "information": "external_custom_id:87123", "phone_numbers": [ { "label": "Work", "value": "+19001112222" } ], "emails": [ { "label": "Office", "value": "gary.jennings@acme.com" } ] }
ResponseStatus: 201 Created { "contact": { "id": 719, "direct_link": "https://api.aircall.io/v1/contacts/719", "first_name": "Gary", "last_name": "Jennings", "company_name": null, "information": "external_custom_id:87123", "is_shared": true, "created_at": 1585083040, "updated_at": 1585083040, "emails": [ { "id": 9170, "label": "Office", "value": "gary.jennings@acme.com" } ], "phone_numbers": [ { "id": 9171, "label": "Work", "value": "+19001112222" } ] } }
Update a shared Contact. Response will be the updated Contact.
To update a Contact's phone numbers or emails, please use the approriate endpoints described below.
This request is a
POST
method, and not a PUT
method!
-
Path params
-
idIntegerUnique identifier for the Contact.
-
Body params
-
first_nameStringCan't be blank if defined.
-
last_nameStringCan't be blank if defined.
-
company_nameStringCan't be blank if defined.
-
informationStringCan't be blank if defined.
RequestPOST /v1/contacts/:id { "first_name": "Vicente UPDATED", "company_name": "Lerox Inc." }
ResponseStatus: 200 OK { "contact": { "id": 710, "direct_link": "https://api.aircall.io/v1/contacts/710", "first_name": "Vicente UPDATED", "last_name": "Abad", "company_name": "Lerox Inc.", "information": null, "is_shared": true, "created_at": 1400691054, "updated_at": 1444336506, "emails": [], "phone_numbers": [ { "id": 3367, "label": "Mobile", "value": "+34664673697" } ] } }
Delete a contact from the Company.
Only shared contacts can be deleted.
-
Path params
-
idIntegerUnique identifier for the Contact.
RequestDELETE /v1/contacts/:id
ResponseStatus: 204 No Content
Phone numbers can be added one by one to a Contact (with a limit of 20, beyond 20 numbers, a 409 error will be returned).
Phone number's value
will be normalized before being stored.
-
Attributes
-
idIntegerUnique identifier for the Contact.
-
Body params
-
labelStringCan't be blank if defined.
-
valueStringAircall stores any phone number format. The number needs to be valid, if not, agents won't be able to dial the contactMandatory field.
RequestPOST /v1/contacts/:id/phone_details { "label": "Work", "value": "+19161110000" }
ResponseStatus: 201 Created { "phone_detail": { "id": 5200, "label": "Work", "value": "19161110000" } }
Phone number's value
will be normalized before being stored.
The value
field must be sent each time a phone number is updated.
-
Attributes
-
idIntegerUnique identifier for the Contact.
-
phone_number_idIntegerUnique identifier for the phone number.
-
Body params
-
labelStringCan't be blank if defined.
-
valueStringAircall stores any phone number format. It is recommended to store a valid phone number so agent can dial them easily.Mandatory field.
RequestPUT /v1/contacts/:id/phone_details/:phone_number_id { "label": "Home UPDATED", "value": "+19161110011" }
ResponseStatus: 202 Accepted { "phone_detail": { "id": 5200, "label": "Home UPDATED", "value": "19161110011" } }
Phone numbers can be removed from a Contact by using the following endpoint.
-
Attributes
-
idIntegerUnique identifier for the Contact.
-
phone_number_idIntegerUnique identifier for the phone number.
RequestDELETE /v1/contacts/:id/phone_details/:phone_number_id
ResponseStatus: 204 No Content
Emails can be added one by one to a Contact (with a limit of 20, beyond 20 emails, a 409 error will be returned).
-
Attributes
-
idIntegerUnique identifier for the Contact.
-
Body params
-
labelStringCan't be blank if defined.
-
valueStringAircall stores any email format.Mandatory field.
RequestPOST /v1/contacts/:id/email_details { "value": "v.lightner@example.com" }
ResponseStatus: 201 Created { "email_detail": { "id": 5201, "label": "Work", "value": "v.lightner@example.com" } }
The value
field must be sent each time an email address is updated.
-
Attributes
-
idIntegerUnique identifier for the Contact.
-
email_idIntegerUnique identifier for the Email address.
-
Body params
-
labelStringCan't be blank if defined.
-
valueStringAircall stores any email format.Mandatory field.
RequestPUT /v1/contacts/:id/email_details/:email_id { "value": "v.updated@example.com" }
ResponseStatus: 202 Accepted { "email_detail": { "id": 5201, "label": "Work", "value": "v.updated@example.com" } }
Email addresses can be removed from a Contact by using the following endpoint.
-
Attributes
-
idIntegerUnique identifier for the Contact.
-
email_idIntegerUnique identifier for the email address.
RequestDELETE /v1/contacts/:id/email_details/:email_id
ResponseStatus: 204 No Content
Tag
Calls can be tagged by Agents from the Phone app.
Tags can be created either by Admins from their Dashboard, or via the Public API, and are made of a name
and a color
.
-
Attributes
-
idIntegerUnique identifier for the Tag.
-
direct_linkStringDirect API URL.
-
nameStringTag's name.
-
colorStringThe color that this tag is displayed in.
-
descriptionStringField used by Aircall to qualify Tags.
Emojis can't be used in Tag's attributes (they will be removed).
Fetch all Tags associated to a company and their information.
Pagination params can be used on this request.
code | description |
---|---|
200 | Success. |
403 | Forbidden. Invalid API key or Bearer access token |
RequestGET /v1/tags
ResponseStatus: 200 OK { "meta": { "count": 3, "total": 3, "current_page": 1, "per_page": 20, "next_page_link": null, "previous_page_link": null }, "tags": [ { "id": 678, "name": "General Inquiries", "color": "#0662B5", "description": null }, { "id": 679, "name": "Feedback", "color": "#008f6c", "description": null }, { "id": 680, "name": "Spam Call", "color": "#FCBB26", "description": null } ] }
Retrieve details of a specific Tag.
-
Path params
-
idIntegerUnique identifier for the Tag.
code | description |
---|---|
200 | Success. |
403 | Forbidden. Invalid API key or Bearer access token |
404 | Not found. Id does not exist |
RequestGET /v1/tags/:id
ResponseStatus: 200 OK { "tag": { "id": 678, "name": "General Inquiries", "color": "#0662B5", "description": null } }
Aircall will perform validation before inserting new Tag (like presence of a name
).
-
Body params
-
nameStringCan't be blank if defined.Mandatory field.The name must be unique.
-
colorStringCan't be blank and must ne formatted in hexadecimalMandatory field.
code | description |
---|---|
201 | Success. |
400 | Invalid payload/Bad Request. |
403 | Forbidden. Invalid API key or Bearer access token |
RequestPOST /v1/tags { "name": "VIP Customer", "color": "#00B388" }
ResponseStatus: 201 Created { "tag": { "id": 681, "name": "VIP Customer", "color": "#00B388", "description": null } }
Some fields can be updated via the Public API.
-
Path params
-
idIntegerUnique identifier for the Tag.
-
Body params
-
nameStringCan't be blank if defined.Mandatory field.The name must be unique.
-
colorStringCan't be blank and must be formatted in Hexadecimal.Mandatory field.
code | description |
---|---|
200 | Success. |
400 | Invalid payload/Bad Request. |
403 | Forbidden. Invalid API key or Bearer access token |
404 | Not found. Id does not exist |
RequestPUT /v1/tags/:id { "name": "Tier 2 Customer" }
ResponseStatus: 200 OK { "tag": { "id": 681, "name": "Tier 2 Customer", "color": "#00B388", "description": null } }
Tag will be removed from Calls.
-
Path params
-
idIntegerUnique identifier for the Tag.
code | description |
---|---|
204 | Success. |
403 | Forbidden. Invalid API key or Bearer access token |
404 | Not found. Id does not exist |
422 | Server unable to process the request, error will be described in the troubleshoot field. |
RequestDELETE /v1/tags/:id
ResponseStatus: 204 No Content
Webhook
This section describes only REST endpoints related to Webhooks.
If you want to know more about Webhook events and how to use them, please refer to this section.
Aircall Public API allows any developer to fetch, create, update and delete Webhooks for an Aircall account.
With OAuth, only the webhook linked to the token
is accessible.
With Basic Auth, webhooks created through the Aircall's dashboard and Basic Auth API requests are accessible.
Webhooks are mainly composed of a custom_name
and a list of events
(see full events list here).
Use the token
field to identify from which Aircall account a Webhook is sent from!
-
Attributes
-
webhook_idstring (uuid)New unique identifier for the Webhook.
-
direct_linkStringDirect API URL.
-
created_atStringTimestamp when the webhook was created, in UTC.
-
custom_nameStringWebhook's custom name.Default is
Webhook
. -
urlStringValid URL to a web server.
-
activeBooleanStatus of the Webhook. If
active
, events will be sent to theurl
.Default istrue
. -
tokenStringUnique token for request's authentication.
-
eventsArrayList of events registered.
Fetch all Webhooks associated to a company and their information.
Old Webhook “Id” can be used to retrieve new “Webhook_id” (UUID).
-
Query params
-
orderStringReorder entries by
created_at
. Can beasc
ordesc
.Default value isasc
Pagination params can be used on this request.
code | description |
---|---|
200 | Success. |
403 | Forbidden. Invalid API key or Bearer access token |
RequestGET /v1/webhooks
ResponseStatus: 200 OK { "meta": { "count": 2, "total": 2, "current_page": 1, "per_page": 20, "next_page_link": null, "previous_page_link": null }, "webhooks": [ { "webhook_id": "c2501111-8a69-4342-bb34-bcd6cfe564ab", "direct_link": "https://api.aircall.io/v1/webhooks/1001", "created_at": "2020-04-01T15:56:18.000Z", "url": "https://my-server.example.com/webhooks/calls", "active": true, "token": "abc123def456ghi789", "events": [ "call.assigned", "call.transferred", "call.ringing_on_agent" ] }, { "webhook_id": "c2501111-8a69-4342-bb34-bcd6cfe564ac", "direct_link": "https://api.aircall.io/v1/webhooks/1002", "created_at": "2020-04-02T09:14:31.000Z", "url": "https://my-server.example.com/webhooks/numbers", "active": true, "token": "4567ghi789abc123def", "events": [ "number.opened", "number.closed" ] } ] }
Retrieve details of a specific Webhook.
-
Path params
-
webhook_idStringUnique identifier for the Webhook.
Old Webhook “Id” can be used to retrieve new “Webhook_id” (UUID).
code | description |
---|---|
200 | Success. |
403 | Forbidden. Invalid API key or Bearer access token |
404 | Not found. Id does not exist |
RequestGET /v1/webhooks/:webhook_id
ResponseStatus: 200 OK { "webhook": { "webhook_id": "c2501111-8a69-4342-bb34-bcd6cfe564ab", "direct_link": "https://api.aircall.io/v1/webhooks/1001", "created_at": "2020-04-01T15:56:18.000Z", "url": "https://my-server.example.com/webhooks/calls", "active": true, "token": "abc123def456ghi789", "events": [ "call.assigned", "call.transferred", "call.ringing_on_agent" ] } }
Webhook creation can be done either from the Aircall Dashboard or via the Public API. Webhook have a list of events attached to it, linked to Calls, Users, Contacts and/or Numbers.
A company can have up to 100 Webhooks maximum.
-
Body params
-
urlStringCan't be blank and must be a valid URL.Mandatory field.
-
custom_nameString
-
eventsArrayIf
events
field is empty, all events will be attached to this webhook.Check here for a full list of available events.
code | description |
---|---|
201 | Success. |
400 | Either url param is missing, company reached their maximum Webhooks count (100) or events are not valid. |
403 | Forbidden. Invalid API key or Bearer access token |
RequestPOST /v1/webhooks { "custom_name": "My Custom Workflow", "url": "https://my-server.example.com/webhooks/contacts", "events": [ "contact.created", "contact.updated", "contact.deleted" ] }
ResponseStatus: 201 Created { "webhook": { "webhook_id": "c2501111-8a69-4342-bb34-bcd6cfe564ab", "direct_link": "https://api.aircall.io/v1/webhooks/26316", "created_at": "2020-03-24T19:51:24.000Z", "url": "https://my-server.example.com/webhooks/contacts", "active": true, "events": [ "contact.created", "contact.updated", "contact.deleted" ], "token": "df76g76dpziygs567f0" } }
Webhook objects can be updated either from the Aircall Dashboard or via the Public API.
This endpoint is also useful to re-activate Webhooks that are automatically disabled by Aircall (more info in the Webhook usage section).
If the
events
field is not specified, Webhook will be registered to all events by default. If you don't want the events
array to be overridden by the default value then please specify query param events_action=add
.
-
Path params
-
webhook_idStringUnique identifier for the Webhook.
Old Webhook “Id” can be used to retrieve new “Webhook_id” (UUID).
-
Query params
-
events_actionStringUse to add or remove specific events from existing list of events.Can be
add
orremove
to Add or Remove Events along withevent name
inevents
array
-
Body params
-
urlStringCan't be blank and must be a valid URL.
-
custom_nameString
-
eventsArrayIf
events
field is empty, all events will be attached to this webhook.Check here for a full list of available events. -
activeBooleanSet this field to
true
to re-activate a Webhook orfalse
to disable it.
code | description |
---|---|
200 | Success. |
400 | Either url param is missing, company reached their maximum Webhooks count (100) or events are not valid. |
403 | Forbidden. Invalid API key or Bearer access token |
404 | Bad request - please provide valid Webhook_id |
RequestPUT /v1/webhooks/:webhook_id { "url": "https://my-new-server.example.com/webhooks/contacts", "events": [ "contact.created", "contact.updated", "contact.deleted" ] }
ResponseStatus: 200 OK { "webhook": { "webhook_id": "c2501111-8a69-4342-bb34-bcd6cfe564ac", "direct_link": "https://api.aircall.io/v1/webhooks/26316", "created_at": "2020-03-24T19:51:24.000Z", "url": "https://my-new-server.example.com/webhooks/contacts", "active": true, "events": [ "contact.created", "contact.updated", "contact.deleted" ], "token": "df76g76dpziygs567f0" } }
Webhook objects can be deleted either from the Aircall Dashboard or via the Public API. Aircall will stop sending events and configuration of the Webhook will be lost.
-
Path params
-
webhook_idStringUnique identifier for the Webhook.
Old Webhook “Id” can be used to retrieve new “Webhook_id” (UUID).
code | description |
---|---|
204 | Success. |
403 | Forbidden. Invalid API key or Bearer access token |
404 | Not found. Id does not exist |
RequestDELETE /v1/webhooks/:webhook_id
ResponseStatus: 204 No Content
Company
Company information can be retrieve with this GET request.
code | description |
---|---|
200 | Success. |
403 | Forbidden. Invalid Bearer access token |
RequestGET /v1/company
ResponseStatus: 200 OK { "company": { "name": "Acme Inc.", "users_count": 146, "numbers_count": 28 } }
Integration
The Integration object is a brief representation of the state of an integration with its name, logo, status, amount of Numbers connected and User that installed it.
Integrations are not updatable nor destroyable via Aircall Public API. The only way to do so is via the Aircall Dashboard.
-
Attributes
-
nameStringName of the Integration.
-
custom_nameStringCustom name of the Integration.
-
logoStringUrl to the Integration logo
-
company_idNumberId of the associated Company
-
statusStringStatus of the integration
-
activeBooleantrue if
status
== "active". -
number_idsNumber[]The ids of Numbers in the Integration.
-
numbers_countNumberNumber of Numbers in the Integration.
-
userUserUser that installed the Integration.Can be
null
if the user was deleted
Integration information can be retrieved with this GET request. The return of this endpoint is the integration associated to the accessToken.
This information can only be retrieved for integrations built by aircall, or 3rd party using OAuth. Integrations using Basic Auth aren’t retrievable through this endpoint.
code | description |
---|---|
200 | Success. |
403 | Forbidden. Invalid Bearer access token |
403 | Forbidden. Cannot be used with API KEY (Oauth only) |
404 | Not found. Integration does not exist anymore |
RequestGET /v1/integrations/me
ResponseStatus: 200 OK { "integration": { "id": 1234, "name": "My app", "logo": "https://cdn.aircall.io/applications/standard/staging/ico_91.svg", "company_id": 1, "status": "active", "active": true, "created_at": "2020-11-02T15:38:16.000+00:00", "updated_at": "2020-11-02T15:38:29.000+00:00", "deleted_at": null, "number_ids": [123456], "numbers_count": 1, "custom_name": null, "user": { "id": 456, "direct_link": "https://api.aircall.io/v1/users/456", "name": "John Doe", "email": "john.doe@aircall.io", "available": true, "availability_status": "available", "created_at": "2019-12-29T10:03:18.000Z", "time_zone": "America/New_York", "language": "en-US" } }, } }
Use this endpoint to enable the integration associated to the access token and activate webhooks on it.
When an integration is first installed, it is enabled by default. This endpoint is handy to programatically re-enable an integration that has been disabled.
If used upon the installation of your integration, with the install true query parameter, this will redirect the user who installed it to your integration settings page in the dashboard at the end of the flow, instead of the aircall integration list. This isn’t mandatory as part of the install flow.
This endpoint can only be used for integrations built by aircall, or 3rd party using OAuth. Integrations using Basic Auth cannot be enabled through this endpoint.
query-params-field | type | description |
---|---|---|
install | Boolean | Pass if first time enabled to see the integration in the dashboard |
code | description |
---|---|
204 | Success. |
403 | Forbidden. Invalid Bearer access token |
403 | Forbidden. Cannot be used with API KEY (Oauth only) |
RequestPOST /v1/integrations/enable
ResponseStatus: 204 OK
Use this endpoint to disable the integration associated to the access token and de-activate webhooks on it.
This endpoint can only be used for integrations built by aircall, or 3rd party using OAuth. Integrations using Basic Auth cannot be disabled through this endpoint.
<!-- URL -->
code | description |
---|---|
204 | Success. |
403 | Forbidden. Invalid Bearer access token |
403 | Forbidden. Cannot be used with API KEY (Oauth only) |
RequestPOST /v1/integrations/disable
ResponseStatus: 204 OK
Participant
The Participant object is a representation of a member in a conference call. A conference call is a call involving more than two participants.
Participant are not updatable nor destroyable via Aircall Public API.
Participant information is only present in conference calls and it can be accessed inside the call object or the conversation intelligence object
Please note
participants
attribute is referred as conference_participants
in Call APIs and as participants
in call Webhook events.
Also, the attributes of Participant Object for Call and Conversation Intelligence Object are different. Please view the Participant Object attributes list for Call and Conversation Intelligence Object below.
Attributes list for Call Object
-
Attributes
-
idStringEither Contact or User id. Not present for external
-
typeStringIt will be 'user', 'contact' or 'external'
-
nameStringParticipant's full name. Not present for external
-
phone_numberStringNot present in a user type participant
Attributes list for Conversation Intelligence Object
-
Attributes
-
participant_typeStringIt will be 'internal' or 'external'.This field is only applicable for transcription.created event
-
phone_numberStringNot present in a 'internal' Participant type
-
valueStringIt will be 'NEUTRAL', 'POSITIVE', or 'NEGATIVE'.This field is only applicable for sentiment.created event
-
user_idStringUnique identifier for the user. Not present in a "external" Participant typeThis field is only applicable for transcription.created event
IVR Option
The IVR Option object is a representation of ivr option selected in smartflow enabled number
IVR object are not updatable or destroyable via Aircall Public API.
IVR information is only present in calls on smartflow enabled numbers and it can be accessed inside the call object.
-
Attributes
-
idStringId of the ivr option selected
-
titleStringTitle of the ivr option selected
-
keyStringKey/Digits pressed by the caller in ivr widgetIf No or Wrong/Invalid Input is selected by customer, then Key value will be
fallback
-
branchStringThe branch name configured in the call distribution
-
created_atStringTimestamp when the IVR option is selected
-
transition_started_atStringTimestamp when the IVR option starts i.e. user starts listening to IVR Options.
-
transition_ended_atStringTimestamp when the IVR option ends i.e. user selects an option or default option is selected.
IVR Object is only available for call done in
last 2 months
from current date
All timestamps are in ISO 8601 format
IVR object is available when adding
fetch_call_timeline
is set to “true” in query parameter for Retrieve a Call, List all calls, Search calls Apis
A2P Campaign association
In order to comply to 10DLC regulation in the US, partners have to associate their Aircall numbers with campaigns previously declared with TCR (The Campaign Registry).
Aircall Public API allows any developer to fetch, create, update and delete A2P campaign associations to numbers. This process involving external APIs of carriers and TCR is executed asynchronously. This mean each API call will be taken into account immediately but those changes will be propagated to the external services after few seconds. A status
column is exposed on the configuration entity to let you know what is the status of the background operation. Following any creation or update action this status
will be pending
and updated to done
once the background operation is completed. In case of error status
will be set to failed
with additional information in the updateMessage
field.
attributes-field | type | description |
---|---|---|
id | Integer | Unique identifier for the A2P campaign association. |
company_id | Integer | Campaign identifier for the A2P campaign association. |
external_id | String | TCR external campaign identifier. |
update_status | String | Status of the asynchronous update, can be pending , done or failed . |
update_message | String | Error message when the updateStatus is failed else null . |
created_at | String | Date when the association was created, in UTC. |
updated_at | Boolean | Date when the association was updated for the last time, in UTC. |
numbers | Array<String> | List of numbers in e.164 format (without + prefix) associated to the A2P campaign. |
EndpointsGET /v1/a2p_campaign_associationsList A2P campaign associations GET /v1/a2p_campaign_associations/:idRetrieve an A2P campaign association POST /v1/a2p_campaign_associationsCreate an A2P campaign association PUT /v1/a2p_campaign_associations/:idUpdate an A2P campaign association DELETE /v1/a2p_campaign_associations/:idDelete an A2P campaign association
Fetch all A2P campaign associations associated to a Company and their information.
Pagination params can be used on this request.
RequestGET /v1/a2p_campaign_associations
ResponseStatus: 200 OK { "meta": { "count": 1, "total": 1, "current_page": 1, "per_page": 20, "next_page_link": null, "previous_page_link": null }, "a2p_campaign_associations": [ { "id": 458, "company_id": 501, "external_id": "CNXIO", "update_status": "pending", "update_message": null, "created_at": "2020-02-18T20:52:22.000Z", "updated_at": "2020-02-18T20:52:22.000Z", "numbers": [ 1279876456 ] } ] }
Retrieve details of a specific A2P campaign association.
-
Path params
-
idIntegerUnique identifier for the A2P campaign association.
RequestGET /v1/a2p_campaign_associations/:id
ResponseStatus: 200 OK { "a2p_campaign_association": { "id": 458, "company_id": 501, "external_id": "CNXIO", "update_status": "pending", "update_message": null, "created_at": "2020-02-18T20:52:22.000Z", "updated_at": "2020-02-18T20:52:22.000Z", "numbers": [ 1279876456 ] } }
A2P campaign association are created via this endpoint to associate Aircall numbers to an external campaign previously declared in TCR. After creation, a unique ID will be included in the response's payload for further actions.
Aircall numbers can be associated to a single 10DLC campaign. Trying to associate a number to multiple campaigns or associating a campaign twice will result in bad request error.
-
Body params
-
external_campaign_idStringTCR external campaign Id.Mandatory field.
-
numbersArray<String>List of numbers in e.164 format (without + prefix) to associate to the A2P campaign.Mandatory field.
code | description |
---|---|
400 | Mandatory attribute missing or incorrect parameters provided, check the troubleshoot field. |
RequestPOST /v1/a2p_campaign_associations { "external_campaign_id": "CNXIO", "numbers": [ 1279876456 ] }
ResponseStatus: 201 Created { "a2p_campaign_association": { "id": 458, "company_id": 501, "external_id": "CNXIO", "update_status": "pending", "update_message": null, "created_at": "2020-02-18T20:52:22.000Z", "updated_at": "2020-02-18T20:52:22.000Z", "numbers": [ 1279876456 ] } }
Aircall numbers associated to an existing A2P campaign association can be updated via this endpoint. The numbers associated will be fully replaced by the one provided in the udpate payload.
Aircall numbers can be associated to a single 10DLC campaign. Trying to associate a number to multiple campaigns via this endpoint will result in bad request error.
-
Path params
-
idIntegerUnique identifier for the A2P campaign association.
-
Body params
-
numbersArray<String>List of numbers in e.164 format (without + prefix) to associate to the existing A2P campaign.Mandatory field.
code | description |
---|---|
400 | Mandatory attribute missing or incorrect parameters provided, check the troubleshoot field. |
RequestPUT /v1/a2p_campaign_associations/:id { "numbers": [ 1279876456 ] }
ResponseStatus: 200 OK { "a2p_campaign_association": { "id": 458, "company_id": 501, "external_id": "CNXIO", "update_status": "pending", "update_message": null, "created_at": "2020-02-18T20:52:22.000Z", "updated_at": "2020-02-18T20:52:22.000Z", "numbers": [ 1279876456 ] } }
Deletes an existing A2P campaign association.
This endpoint deletes the association of Aircall numbers to the A2P external campaign but does not modify the campaign itself.
-
Path params
-
idIntegerUnique identifier for the A2P campaign association.
code | description |
---|---|
204 | A2P campaign association is going to be deleted. |
400 | Mandatory attribute missing or incorrect parameters provided, check the troubleshoot field. |
RequestDELETE /v1/a2p_campaign_associations/:id
ResponseStatus: 204 No Content
Webhooks
Webhooks allow Aircall to provide and send real-time information to other applications.
Aircall Webhooks are designed to notify external system each time an event occurs on an Aircall account, like when a call is started, when a user is created and even when a contact is updated!
With that in mind, any developer can build automated tasks, specific to any businesses, based on these events.
User's events
user.created
user.deleted
user.connected
user.disconnected
user.opened
user.closed
user.wut_start
user.wut_end
Number's events
Message's events
Call's events
call.created
call.ringing_on_agent
call.agent_declined
call.answered
call.transferred
call.external_transferred
call.unsuccessful_transfer
call.hungup
call.ended
call.voicemail_left
call.assigned
call.archived
call.tagged
call.untagged
call.commented
call.hold
call.unhold
call.ivr_option_selected
call.comm_assets_generated
Contact's events
Conversation Intelligence's events
Click on an event's name to get more information on how and when it is trigger by Aircall!
Register an endpoint
The following steps describe how to register a server endpoint to start receiving Webhook events. Up to 100 Webhooks can be registered per account!
- Login on your Aircall account (sign up here if you don't have one).
- Go to the Integrations' page.
- In the Discover more integrations section, find and click on the Webhook block.
- Create a Webhook by clicking on the green Install button.
- Provide a name and a valid URL and select all the events you want to subscribe to.
- Click on the green Save button and… that's it!
Your Webhook URL must be behind a SSL certificate and start with
https
.
Aircall will start sending events to your server right after the Webhook creation, make sure your server is up and running.
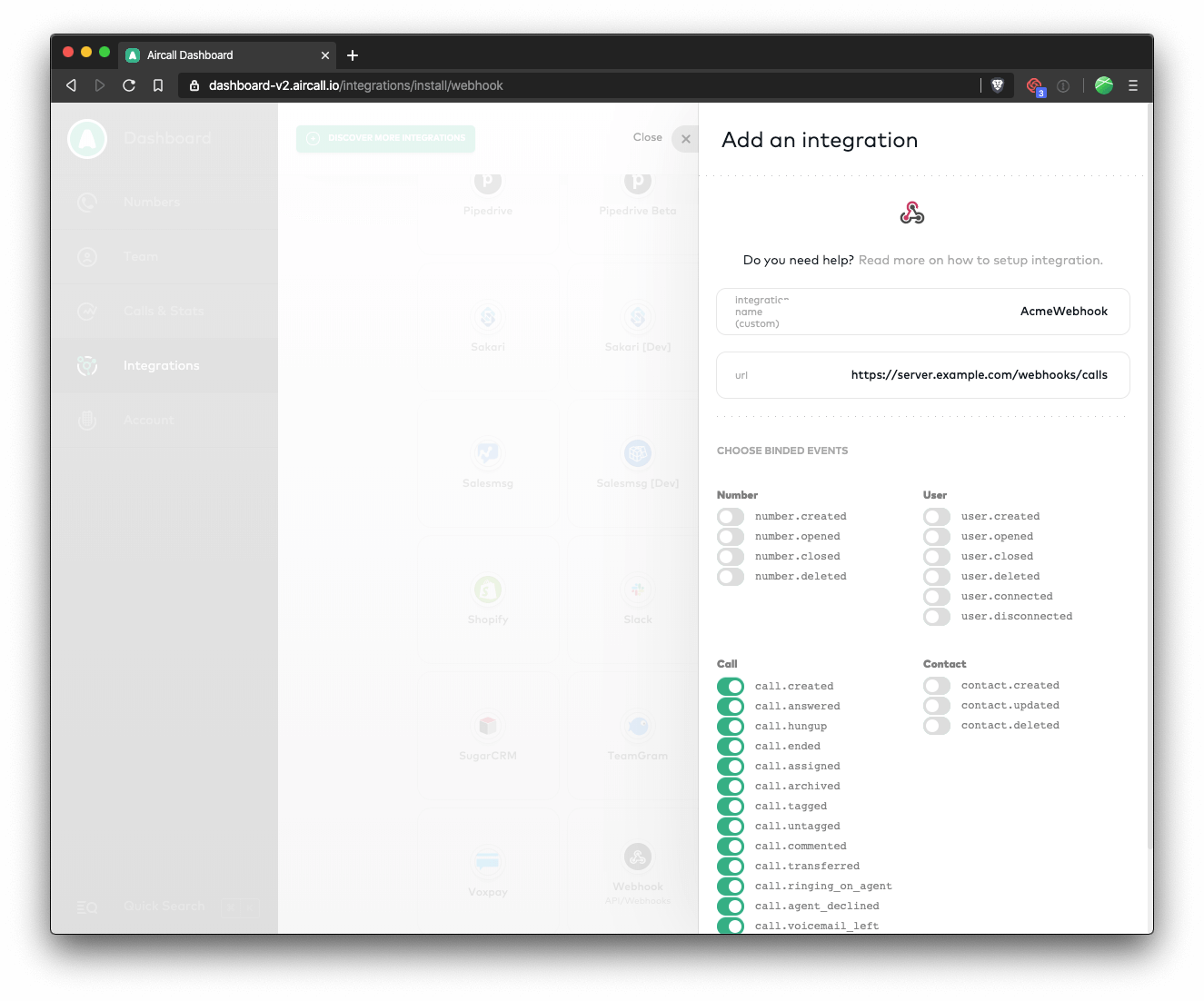
Webhook usage
Make sure your server can receive
POST
requests, reads JSON format and always answers a 200
HTTP Code!
During development, you can create SSH tunnels to your local environment. At Aircall, we use and recommand ngrok which is free and open-source.
Web server
Once the endpoints are registered, a simple web server is needed in order to receive events.
Then, Aircall will send a POST
request to the Webhook's URL each time an event occurs with a JSON payload body.
The Web server must be publicly available, Aircall does not provide a list of static IP addresses to whitelist.
Please note an event will be delivered at least once , if generated, but events might not be delivered in a specific sequence/order
Automatic deactivation
For failed events, Aircall will perform fifty (50) retries and post that if the issue still persists webhook will be disabled and no new events will be sent. An alert on Aircall Dashboard will also indicate webhook being disabled.
Once Webhook is disabled, Aircall will retry only failed events for next 12 hours. In case, a success message is received for failed event(s) the webhook will be automatically enabled.
Admin users can receive email notification regarding webhook being disabled or enabled by enabling email notification via user settings in Aircall dashboard.
A request is considered as failed if the HTTP Status Code answered is not 2XX
or if the request times out.
Make sure your server always answers a 200
HTTP Code, that will prevent Aircall Webhooks from being deactivated!
Aircall's HTTP requests sent to external web servers are set up to timeout after 5 seconds.
Filtering by Numbers
If the integration is built using the OAuth authentication method, Admins will be able to filter from which Numbers they want to receive Call events from on their Aircall Dashboard.
If the integration is using the Basic Auth method, Call webhook events will be sent for all Numbers of a Company.
Payload
Webhook events are sent with a JSON payload attached.
This payload mixes the JSON formatting of an Aircall's object (User, Number, Call, Contact) and some metadata like an absolute timestamp or the name of the event.
-
Webhook attributes
-
resourceStringName of the resource for this event.Can be
number
,user
,contact
orcall
. -
eventStringEvent's name for this payload.See the complete list here.
-
timestampIntegerUNIX timestamp for when the payload was built, in UTC.
-
tokenStringToken associated to the Webhook. Use it to identify from which Aircall account a Webhook event is sent from.
-
dataObjectModelization of the
resource
at thetimestamp
value.
Payload{ "resource": "number", "event": "number.closed", "timestamp": 1585001020, "token": "45XXYYZZa08", "data": { "id": 456, "direct_link": "https://api.aircall.io/v1/numbers/123", "name": "My first Aircall Number", "digits": "+33 1 76 36 06 95", "country": "FR", "time_zone": "Europe/Paris", "open": false, "users": [ { "id": 456, "direct_link": "https://api.aircall.io/v1/users/456", "name": "Madelaine Dupont", "email": "madelaine.dupont@aircall.io", "available": false, "language": "en-US" } ] } }
User events
Sent when new users are invited in an Aircall company.s
Payload{ "event": "user.created", "resource": "user", "timestamp": 1585601319, "token": "45XXYYZZa08", "data": { // the User object } }
Sent when users are deleted from an Aircall company by Admin users.
Payload{ "event": "user.deleted", "resource": "user", "timestamp": 1589609314, "token": "45XXYYZZa08", "data": { // the User object } }
Sent when users open their Aircall Phone.
Payload{ "event": "user.connected", "resource": "user", "timestamp": 158555000, "token": "45XXYYZZa08", "data": { // the User object } }
Sent when users close their Aircall Phone.
Payload{ "event": "user.disconnected", "resource": "user", "timestamp": 1585603000, "token": "45XXYYZZa08", "data": { // the User object } }
Sent when users become available according to their working hours.
Payload{ "event": "user.opened", "resource": "user", "timestamp": 158556187, "token": "45XXYYZZa08", "data": { // the User object } }
Sent when users become unavailable according to their working hours.
For receiving substatus or unavailablity reason selected by a user on Phone App in
user.closed
event, please reach out to Aircall support team at support.aircall.io to enable it for your company. Also, please note that this will result in two user.closed
events being sent to you i.e. 1st one with just availability_status and 2nd one with substatus as selected by user in Phone App. So please ensure to handle the two events at your end and not to treat them as duplicate events.
Payload{ "event": "user.closed", "resource": "user", "timestamp": 158554819, "token": "45XXYYZZa08", "data": { // the User object } }
Sent when user start their wrap up time (WUT) work according to their setting.
If wrap up time is set as zero and have mandatory call tagging enabled,
user.wut_start
event will be sent if user tags the call at the end of the call. However if call was tagged during the call(having mandatory call tagging enabled), the event would not be sent.
Payload{ "event": "user.wut_start", "resource": "user", "timestamp": 158554819, "token": "45XXYYZZa08", "data": { // the User object } }
Sent when user finishes their wrap up time (WUT) work according to their setting.
If wrap up time is set as zero and have mandatory call tagging enabled,
user.wut_end
event will be sent if user tags the call at the end of the call. However if call was tagged during the call(having mandatory call tagging enabled), the event would not be sent.
Payload{ "event": "user.wut_end", "resource": "user", "timestamp": 158554819, "token": "45XXYYZZa08", "data": { // the User object } }
Call events
The following events refers to the Call object.
If your app is using the OAuth authentication method, Admins will be able to filter from which Numbers they want to receive Call events from on their Aircall Dashboard.
If your app is using the Basic Auth method, Call webhook events will be sent for all Numbers of a Company. You can implement this logic on your server!
Inbound calls
Here is a diagram showing when call events are being triggered during inbound calls:
Outbound calls
Here is a diagram showing when call events are being triggered during outbound calls:
Please note following attributes has different naming conventions in Call APIs and Webhook events :
i. sid
and call_uuid
- Both refers to same value but in call APIs attribute is called sid
and in case of call events its called call_uuid
.
ii. participants
and conference_participants
- Both refers to same value but in call APIs attribute is called conference_participants
and in case of call events its called participants
.
Payload{ "resource": "call", "event": "call.created", "timestamp": 1732622896, "token": "cebcaac65XXXXXXXXXx", "data": { "id": 123, "direct_link": "https://api.aircall.io/v1/calls/123", "direction": "outbound", "call_uuid": "CAed058bba60a84d62c77cee898a852b05", "status": "initial", "missed_call_reason": null, "started_at": 1732622895, "answered_at": null, "ended_at": null, "duration": 0, "cost": "0", "hangup_cause": null, "voicemail": null, "voicemail_short_url": null, "recording": null, "recording_short_url": null, "asset": null, "raw_digits": "+1 800-123-4567", "participants":[ { "phone_number" : "+1 800-123-4567" "type" : "external" }, { "phone_number" : "+3512345678" "type" : "external" }, { "id" : 123, "name": "John Doe", "type" : "user" } ], "user": { //the User Object }, "contact": { //the Contact Object }, "number": { //the Number Object } } }
Sent when a call is started.
- For inbound calls, this event is sent when calls hits an Aircall number.
- For outbound calls, this event is sent when Agents start a call from their Phone.
Please note in an IVR flow, customer should link an Integration to either Parent or Child number in IVR Widget to receive only one call.created event. If Child number is linked to Integration then please reach out to Aircall Support team on support.aircall.io to inform and get IVR Call redirection enabled for Child number.
Linking both Parent and Child will result in duplicate call.created event generated for each number and should be handled by customer at their end.
Payload{ "event": "call.created", "resource": "call", "timestamp": 1585001000, "token": "45XXYYZZa08", "data": { // the Call object } }
Sent when calls start ringing on Agents' Phone.
Payload{ "event": "call.ringing_on_agent", "resource": "call", "timestamp": 1585001010, "token": "45XXYYZZa08", "data": { // the Call object } }
Sent when Agents decline inbound calls.
Payload{ "event": "call.agent_declined", "resource": "call", "timestamp": 1585001020, "token": "45XXYYZZa08", "data": { // the Call object } }
For inbound calls, this event is sent only when one Agent picks the call up.
For outbound calls, this event is sent only when the external person picks the call up.
The call
direction
is in the data
object present in the payload.
Payload{ "event": "call.answered", "resource": "call", "timestamp": 1585001020, "token": "45XXYYZZa08", "data": { // the Call object } }
Calls can be transferred either by Agents from their Aircall Phone, or via the Public API (see here).
- When calls are transferred to another Agent, this event is sent when the other agent answers the call.
- When calls are transferred to a Team, this event is sent when one agent answers the call.
- When calls are transferred to an external number via the Aircall Phone, this event is not sent.
- When calls are transferred to an external number via the Public API, this event is sent without the transferred_to field in the payload.
If the external party does not answer the call,
call.transferred
event will not be sent and call will return back to the original agent.
More details on Transferring calls in our Knowledge Base.
Payload{ "event": "call.transferred", "resource": "call", "timestamp": 1585001030, "token": "45XXYYZZa08", "data": { // the Call object } }
Calls can be transferred to external number by Agents from their Aircall Phone.
- When calls are transferred to an external number via the Aircall Phone, this event is sent when external agent answers the call.
- When calls are transferred to an external number via the Aircall Phone, this event is sent without the
transferred_to
field in the payload, instead it will have a new fieldexternal_transferred_to
which will be a string indicating the external number.
If the external party does not answer the call,
call.external_transferred
event will not be sent and call will return back to the original agent.
Payload{ "event": "call.external_transferred", "resource": "call", "timestamp": 1585001030, "token": "45XXYYZZa08", "data": { // the Call object "external_transferred_to": "919205152487", //New field } }
Calls can be transferred either by Agents from their Aircall Phone, or via the Public API (see here).
- For calls that are unsuccessfully transferred to another Agent, this event is sent when the other agent doesn't answer the call.
- For calls that are unsuccessfully transferred to a Team, this event is sent when no agent answers the call.
- For calls that are unsuccessfully transferred to an external number via the Aircall Phone, this event is not sent.
- For calls that are unsuccessfully transferred to an external number via the Public API, this event is sent when the external line is busy and without the transferred_to field in the payload.
More details on Transferring calls in our Knowledge Base.
Payload{ "event": "call.unsuccessful_transfer", "resource": "call", "timestamp": 1585001030, "token": "45XXYYZZa08", "data": { // the Call object } }
Sent immediately after calls are hung up.
Some fields present in the payload body might be null as Aircall needs ~30sec to gather extra data like the duration and recording files.
This event must only be used to know when a call is hung up whereas
call.ended
and call.voicemail_left
can be used to retrieve extra data such as the recording file, call duration etc.
Payload{ "event": "call.hungup", "resource": "call", "timestamp": 1585001040, "token": "45XXYYZZa08", "data": { // the Call object } }
Sent ~30sec after calls are actually hung up as Aircall server needs time to gather and process extra data like duration and recording files.
This event must only be used to retrieve all calls information whereas
call.hungup
can be used to know in real time when a call is ended.
Payload{ "event": "call.ended", "resource": "call", "timestamp": 1585002050, "token": "45XXYYZZa08", "data": { // the Call object } }
Sent ~30sec after calls end if a voicemail is present. Voicemail file will be included in the data
object under the voicemail
and asset
fields.
Payload{ "event": "call.voicemail_left", "resource": "call", "timestamp": 1585002050, "token": "45XXYYZZa08", "data": { // the Call object } }
Calls can be commented, either by Agents from their Aircall Phone, or via the Public API (see here).
This event is sent when this action is performed and a new comment is created on a Call.
If the call is ongoing and the action is done by the agent in the in-call view, this event will be triggered at the end of the call.
Payload{ "event": "call.commented", "resource": "call", "timestamp": 1585601319, "token": "45XXYYZZa08", "data": { // the Call object } }
Calls can be tagged either by Agents from their Aircall Phone, or via the Public API (see here).
This event is sent when this action is performed.
If the call is ongoing and the action is done by the agent in the in-call view, this event will be triggered at the end of the call.
Payload{ "event": "call.tagged", "resource": "call", "timestamp": 1585601319, "token": "45XXYYZZa08", "data": { // the Call object } }
Tags can be removed from calls by Agents from their Aircall Phone.
This event is sent when this action is performed and does not apply on ongoing calls.
Payload{ "event": "call.untagged", "resource": "call", "timestamp": 1585601319, "token": "45XXYYZZa08", "data": { // the Call object } }
Calls can be assigned to an Agent by another Agent, from the Aircall Phone.
This event is sent when this action is performed.
Payload{ "event": "call.assigned", "resource": "call", "timestamp": 1585601319, "token": "45XXYYZZa08", "data": { // the Call object } }
Calls can be archived (=mark as done) either by Agents from their Aircall Phone, or via the Public API (see here).
This event is sent when this action is performed.
Payload{ "event": "call.archived", "resource": "call", "timestamp": 1585601319, "token": "45XXYYZZa08", "data": { // the Call object } }
Sent when a call is put on hold.
Payload{ "event": "call.hold", "resource": "call", "timestamp": 1585001000, "token": "45XXYYZZa08", "data": { // the Call object } }
Sent when a call is removed from on hold.
Payload{ "event": "call.unhold", "resource": "call", "timestamp": 1585001000, "token": "45XXYYZZa08", "data": { // the Call object } }
Sent after ivr option is selected on a smartflow enabled number.
This event must only be used to know when a ivr option is selected on a smartflow enabled number.
Payload{ "event": "call.ivr_option_selected", "resource": "call", "timestamp": 1585001040, "token": "45XXYYZZa08", "data": { // the Call object } }
Event is sent when communication assets for a call i.e. call recording or voicemail is generated. Only one event per call is sent. This event can be referred if call recording or voicemail link is not available in call.ended event.
This event will be sent only when a call is recorded or a voicemail is left.
Payload{ "event": "call.comm_assets_generated", "resource": "call", "timestamp": 1585001040, "token": "45XXYYZZa08", "data": { // the Call object } }
Number events
The following events refers to the Number object.
Numbers can be bought and deleted by Aircall Admin users.
Sent when new numbers are created on Aircall accounts.
Payload{ "event": "number.created", "resource": "number", "timestamp": 1585601319, "token": "45XXYYZZa08", "data": { // the Number object } }
Sent when numbers are deleted from Aircall accounts by Admin users.
Payload{ "event": "number.deleted", "resource": "number", "timestamp": 1589609314, "token": "45XXYYZZa08", "data": { // the Number object } }
Sent when a number opens according to its opening hours.
Payload{ "event": "number.opened", "resource": "number", "timestamp": 158556187, "token": "45XXYYZZa08", "data": { // the Number object } }
Sent when a number closes according to its opening hours.
Payload{ "event": "number.closed", "resource": "number", "timestamp": 158554819, "token": "45XXYYZZa08", "data": { // the Number object } }
Message events
The following events refers to the Message object.
Messages can be sent to or received from Aircall numbers.
Inbound message
Here is a diagram showing when message events are being triggered during inbound message:
Outbound message
Here is a diagram showing when message events are being triggered during outbound message:
Sent when a new message is sent from Aircall accounts.
Payload{ "event": "message.sent", "resource": "message", "timestamp": 1585601319, "token": "45XXYYZZa08", "data": { // the Message object // the Number object // the Contact object } }
Sent when a new message is received to Aircall accounts.
Payload{ "event": "message.received", "resource": "message", "timestamp": 1589609314, "token": "45XXYYZZa08", "data": { // the Message object // the Number object // the Contact object } }
Sent when the message from Aircall accounts has an updated status.
Payload{ "event": "message.status_updated", "resource": "message", "timestamp": 158556187, "token": "45XXYYZZa08", "data": { // the Message object // the Number object // the Contact object } }
Contact events
Sent when new contacts are created on an Aircall account.
Payload{ "event": "contact.created", "resource": "contact", "timestamp": 1585601319, "token": "45XXYYZZa08", "data": { // the Contact object } }
Sent when new contacts are updated on an Aircall account.
Payload{ "event": "contact.updated", "resource": "contact", "timestamp": 158561587, "token": "45XXYYZZa08", "data": { // the Contact object } }
Sent when new contacts are deleted on an Aircall account.
Payload{ "event": "contact.deleted", "resource": "contact", "timestamp": 158562010, "token": "45XXYYZZa08", "data": { // the Contact object } }
Integration events
The following events refer to the Integration object.
Integrations can be created and deleted by Aircall Admin users.
Sent when an integration is deleted. This event is only sent for webhooks created by applications that use Aircall OAuth credentials.
This event is useful for technology partners, to make sure uninstall flows are synced between Aircall and their application. When the integration is deleted in Aircall, we recommend deleting it on your side as well.
Payload{ "event": "integration.deleted", "resource": "integration", "timestamp": 158554819, "token": "45XXYYZZa08", "data": { "integration_id": 42, "company_id": 1 } }
Conversation Intelligence events
The following events refers to the Conversation Intelligence object.
Conversation Intelligence Events are AI-generated events related to AI entities such as Call Summary, Key Topics, Call Transcription, Sentiment Analysis etc.
Sent after Sentiment Analysis is generated for a Call and indicates customer’s sentiment/mood on the call.
This event is only generated when a company has signed up for Aircall AI package
Payload{ "event": "sentiment.created", "resource": "conversation_intelligence", "timestamp": 1585001000, "token": "45XXYYZZa08", "data": { // the Conversation Intelligence object } }
Sent after call summary is generated for a call.
This event is only generated when a company has signed up for Aircall AI package
Payload{ "event": "summary.created", "resource": "conversation_intelligence", "timestamp": 1585001000, "token": "45XXYYZZa08", "data": { // the Conversation Intelligence object } }
Sent after Key topics for a Call are generated.
This event is only generated when a company has signed up for Aircall AI package
Payload{ "event": "topics.created", "resource": "conversation_intelligence", "timestamp": 1585001000, "token": "45XXYYZZa08", "data": { // the Conversation Intelligence object } }
Sent after Transcription is generated for a Call.
Transcription wont be available via transcription.created event. It will only notify availability of Transcription for a call. Please use Retrieve a Transcription API to fetch the Transcription for specific Call.
This event is only generated when a company has signed up for Aircall AI package
Payload{ "event": "transcription.created", "resource": "conversation_intelligence", "timestamp": 1585001000, "token": "45XXYYZZa08", "data": { // the Conversation Intelligence object } }
Changelog
There's only one version of our Public APIs and Webhooks. This changelog is here to present fields and endpoints that have been added overtime.
-
Versions
-
1.59.02025-02-27Add uniqueness validation on Tag Name attribute in Create a Tag and Update a Tag APIs
-
1.58.02025-02-18Add call.external_transferred Webhook event
-
1.57.02025-01-27Remove limit on number of users per team in call transfers
-
1.56.02024-12-18Added warnings to clarify that Smartflows music and messages cannot currently be updated via the Public API.
-
1.55.02024-12-10Clarify error 400 when multiple transfer destinies are set in call transfers
-
1.54.02024-12-10
-
1.53.02024-11-25Add Send message within agent conversation endpoint to Message section.
-
1.52.02024-11-21Forbid multiple transfer destinies in call transfers
-
1.51.02024-11-21
-
1.50.02024-10-24Add summary.created and topics.created Webhook event
-
1.49.02024-10-07Add sid in call response for Call object. Allow to retrieve IVR options on Retrieve a Call, List all calls, Search calls
-
1.48.02024-09-13Add Retrieve a transcription, Retrieve sentiments, Retrieve a summary, Retrieve topics endpoints to Conversation Intelligence section.
-
1.47.02024-09-06
-
1.46.02024-08-26Add transcription.created Webhook event
-
1.45.02024-08-14Add sentiment.created Webhook event
-
1.44.02024-08-07Update Call Id Data Type to Int64 in Call.
-
1.43.02024-08-05Add Create Number configuration, Fetch Number configuration, Delete Number configuration and Send Message endpoints to Message section.
-
1.42.02024-07-29Deprecate
cost
attribute in Call. -
1.41.02024-07-26Add
recording_short_url
andvoicemail_short_url
attributes in Call object for Call retrieve, list and search endpoints when usingfetch_short_urls=true
query parameter. -
1.40.02024-07-10Add
substatus
attribute to User object for Webhook events. -
1.39.02024-06-18Add Query Params
events_action
to Update Webook endpoint. -
1.38.02024-06-03
-
1.37.02024-05-24
-
1.36.02024-05-15Add
[GET] /v1/numbers/:id/registration_status
to Number endpoints. -
1.35.02024-04-29Deprecate
id
parameter in the Webhook endpoint. -
1.34.02024-04-04Deprecate
isAdmin
parameter in the Create a User endpoint. -
1.33.02024-03-25
-
1.32.02024-03-04
-
1.31.02023-11-02Update of Webhook Automatic deactivation Process.
-
1.30.02023-09-28Change call history window time from 6 months to 3 months.
-
1.29.02023-09-19Add
wrap_up_time
input parameter for User's Create and Update methods. -
1.28.12023-07-13Add Information regarding using Number API with Smartflow enabled Numbers.
-
1.28.02023-05-17Introduce a new property
webhook_id
for Webhook endpoints. -
1.27.02022-09-30
-
1.26.02021-09-22
-
1.25.02021-09-27When a call is transferred to an external number via the Public API, transfer Webhook events are now fired.
-
1.24.02021-09-20
-
1.23.02021-09-15
-
1.22.02021-07-30Add
priority
parameter on Number. This property is used during routing to dequeue the agent's tasks. Can benull
,0
(no priority) or1
(top priority). Defaultnull
-
1.21.02021-08-17Update the endpoint
[POST] /v1/contacts
,phone_numbers
parameter is mandatory to create a contact. -
1.20.02021-06-14Add
transferred_by
parameter on Call -
1.19.02021-04-28Add
call.unsuccessful_transfer
event Webhook -
1.18.02021-03-31
-
1.17.02021-02-18Add
integration.deleted
Webhook event. -
1.16.02021-02-17Add Participants to
[GET] v1/calls/:id
endpoint. The participant is a representation of a member in a conference call. -
1.15.02021-01-12Add
dispatching_strategy
parameter on Call transfer. -
1.14.02020-11-26Add User to
[GET] v1/integrations/me
endpoint. The user is the one that installed the integration. -
1.13.02020-11-26Add
language
attribute on User object. -
1.12.22020-11-20When an inbound call is anonymous, the raw_digits value is
anonymous
. -
1.12.22020-11-10Scope the webhook access: it is now only possible to list, update and delete webhooks linked to the request credentials.
-
1.12.12020-11-04Update the endpoint
[DELETE] v1/calls/:id/recording
, it takes between 10 and 15 minutes to delete a call'srecording
from our servers. -
1.12.02020-10-02Add
time_zone
parameter on User object. -
1.11.32020-09-16
-
1.11.22020-06-22Add a
user
type to the Insight Card model. -
1.11.12020-04-15When call is ringing, transfering to a team is now limited to teams with 25 users or less.Transfering to a user or after call as been answered is unchanged.
-
1.11.02020-04-14New documentation design for this API References! 🎉
-
1.10.12019-11-21Deprecate the
[POST] /v1/calls/:id/metadata
and[POST] /v1/calls/:id/link
endpoints, available on the Call object. -
1.10.02019-04-08
-
1.9.12019-02-08Add
number
parameter for external call transfers via Public API. -
1.9.02019-01-10Add
[DELETE] /v1/teams/:id
endpoint on Team object. -
1.8.92018-11-26
-
1.8.82018-11-07Add
cost
parameter on Call object. -
1.8.72018-07-24Add
call.voicemail_left
Webhook event. -
1.8.62018-07-09Add
[DELETE] /v1/users/:id
endpoint on User object. -
1.8.52018-06-27Add
live_recording_activated
to Number. -
1.8.42018-06-27Add
is_shared
attribute to Contact object. -
1.8.32018-06-26New
[POST] /v1/users/:id/dial
endpoint for User object. -
1.8.22018-06-15
-
1.8.12018-05-31Add
call.agent_declined
Webhook event. -
1.8.02018-05-30Add
call.ringing_on_agent
Webhook event. -
1.7.92018-05-25New
[POST] /v1/users/:id/calls
endpoint for User object. -
1.7.82018-05-16Add
asset
attribute to the Call object. -
1.7.72018-04-25New
[POST] /v1/calls/:id/pause_recording
and[POST] /v1/calls/:id/resume_recording
endpoints for Call object. -
1.7.62018-04-03New
[POST] /v1/calls/:id/tags
endpoint for Tags. -
1.7.52018-04-02Add Tag actions via Public API.
-
1.7.42018-03-20Allow
user_id
andphone_number
query params on[GET] /v1/calls/search
search endpoint. Addcall.transferred
Webhook event. -
1.7.32018-01-11
-
1.7.22017-12-16
-
1.7.12017-12-15New
[POST] /v1/calls/:id/comments
route to add a comment to a call. -
1.7.02017-12-05Add Team creation via Public API.
-
1.6.02017-11-22Add User creation via Public API.
-
1.5.02017-10-02Add Webhooks management via Public API.
-
1.4.42017-09-18Add possibility to update User's & Number's name.
-
1.4.32017-09-15Add
call.hungup
event to Webhooks object. -
1.4.22017-09-06Add
is_ivr
parameter on Number object. -
1.4.12017-07-21Add
order_by
parameter on Contact list. -
1.4.02017-03-07
-
1.3.02017-01-16Add
user.connected
anduser.disconnected
events for Webhooks. -
1.2.02016-11-08Add transfers endpoint.
-
1.1.72016-10-23Add search by tags on Call list.
-
1.1.62016-05-23Add
information
field on Contact object. -
1.1.52016-05-02Add tags in Call object.
-
1.1.32015-10-08Add
call.answered
event for Webhooks. -
1.1.22015-10-01Add two timestamps in Call object.
-
1.1.12015-09-28Add recording URL in Call object.
-
1.1.02015-08-03API public release.
-
1.0.92015-07-31API beta release.
-
1.0.42015-07-30Add comments to Call object.
-
1.0.32015-07-29Add assignment to Call object.
-
1.0.22015-07-28Introducing direct API links.
-
1.0.12015-07-27Update Contact object.
-
1.0.02015-06-02Public release.
-
0.9.02015-05-25Beta release.
-
0.1.02015-05-18Alpha release.
-
0.0.12015-05-10🎉 Initial release.
Extras
In this section, we will describe a few non-essential things to know when working with Aircall Public API, like Timezones, Phone numbers formats and emojis.
CTI - Aircall Everywhere SDK
Looking to build a more advanced integration with Aircall? Aircall Everywhere is a JavaScript SDK that lets you embed and control the Phone app in your website.
The Phone app will be loaded in a HTML iframe and will send events to your main page when incoming calls are ringing, when calls are answered, when agents log in... You can also send instructions to the Phone app such as dial a number or exit the keyboard! The full list of events is available on GitHub.
A demo website is available here and the full documentation of it is available on GitHub!
import AircallPhone from 'aircall-everywhere';
const aircallPhone = new AircallPhone({
onLogin: settings => {
console.log('phone loaded');
doStuff();
},
onLogout: () => {},
domToLoadPhone: '#phone',
integrationToLoad: 'zendesk',
size: 'big'
});
Dealing with phone numbers
Aircall uses international phone number formatting in its Public API.
But dealing with phone number formatting can be complex and time consuming. We recommend using libphonenumber, built by Google, complete and up-to-date!
It extracts useful information from any phone number, like the number's type (mobile, toll-free, landline...), the country, the international/national/e164 format...
SIP trunking
Aircall uses several providers under the hood to buy and configure phone numbers all around the World.
As each provider is specific, Aircall does not offer SIP trunking capabilities or any advanced voice feature via its Public API yet!
The best way to get access to the audio data is to get the recording
mp3 file URL at the end of a Call.
Timezones
Aircall dates and times are rendered in UTC.
Timezones listed in Aircall's products follow the tz database format.
Emojis
We love emojis, but they don't fit well everywhere in our products (yet!). You can display emojis in Insight Cards, to make caller insights displayed on the phone more compelling 💅 . For the rest, the Public API will return an error if you try to update any string with an emoji 😱